How to declare a data type in Python?
How to declare a data type in Python?
I'd be happy to help!
Declaring Data Types in Python: A Comprehensive Guide
Python is a dynamic language, which means it doesn't require explicit declaration of variable types at the time of creation. However, as your codebase grows and you need to ensure data integrity, declaring data types can become crucial for readability, maintainability, and performance. In this article, we'll explore how to declare data types in Python.
Implicit Typing
Python is known for its implicit typing system. When you assign a value to a variable without explicitly defining its type, Python infers the type based on the assigned value. For example:
x = 5 # int
y = "hello" # str
z = [1, 2, 3] # list
In this example, x
is implicitly declared as an integer (int
), y
as a string (str
), and z
as a list (list
). Python automatically infers the data type based on the assigned value.
Explicit Typing with Type Hints
While implicit typing is convenient, explicit typing with type hints provides better code readability and helps catch potential errors earlier. Python 3.5 introduced type hints, which allow you to specify the expected data type for a variable or function parameter.
To declare a data type explicitly using type hints:
from typing import List
x: int = 5 # int
y: str = "hello" # str
z: List[int] = [1, 2, 3] # list of ints
Using the type()
Function
Another way to declare a data type is by using the type()
function. This method returns the type of an object. For example:
x = 5 # int
y = "hello" # str
z = [1, 2, 3] # list
print(type(x)) # prints: <class 'int'>
print(type(y)) # prints: <class 'str'>
print(type(z)) # prints: <class 'list'>
Benefits of Declaring Data Types
Declaring data types in Python has several benefits:
Improved Code Readability: Explicitly declaring data types makes your code more readable, as the type information is clearly stated. Better Error Messages: When you encounter an error, explicit typing helps you identify the issue earlier by providing more informative error messages. Improved Code Maintenance: With explicit typing, it's easier to maintain and update your codebase over time.Conclusion
In this article, we explored how to declare data types in Python, including implicit typing, explicit typing with type hints, and using the type()
function. Declaring data types can improve code readability, error messages, and maintenance. By incorporating these techniques into your coding practice, you'll write more efficient, maintainable, and reliable Python code.
Thanks for reading!
Built-in data types in Python
I'm happy to respond in English this time!
In Python, built-in data types refer to the basic data structures that are inherently defined within the language itself. These data types can be used directly without needing to import any external libraries or modules. Here are some of the most commonly used built-in data types in Python:
Integers (int): Integers are whole numbers, either positive, negative, or zero, with no decimal points. For example: 123, -456, or 0.a = 123
print(type(a)) # Output: <class 'int'>
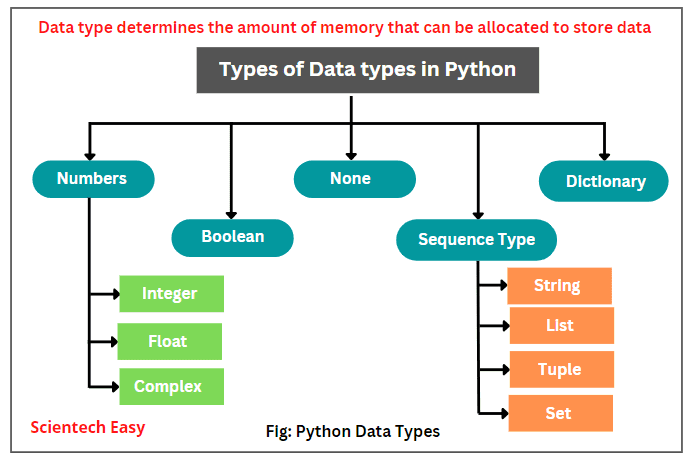
Floats (float): Floats are decimal numbers, which can have a fractional part. For instance: 3.14, -0.5, or 12.0.
b = 3.14
print(type(b)) # Output: <class 'float'>
Strings (str): Strings are sequences of characters, such as words, phrases, or sentences. They can be enclosed in single quotes (''), double quotes (""), or triple quotes (''' or """).
c = 'hello'
print(type(c)) # Output: <class 'str'>
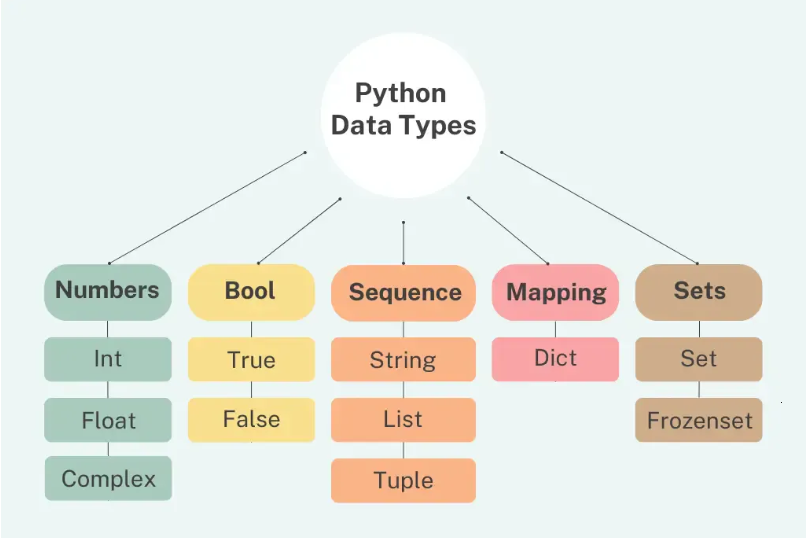
Boolean (bool): Booleans are logical values that can take on two possible states: True
or False
.
d = True
print(type(d)) # Output: <class 'bool'>
List (list): Lists are ordered collections of items, which can be homogeneous (i.e., all elements have the same data type) or heterogeneous (i.e., elements have different data types). They are denoted by square brackets []
.
e = [1, 2, 3]
print(type(e)) # Output: <class 'list'>
Tuple (tuple): Tuples are ordered and immutable collections of items, similar to lists but with the difference that they cannot be modified after creation. They are denoted by round brackets ()
.
f = (1, 2, 3)
print(type(f)) # Output: <class 'tuple'>
Dictionary (dict): Dictionaries are unordered collections of key-value pairs, often used to represent data structures like hash maps or associative arrays. They are denoted by curly braces {}
.
g = {'name': 'John', 'age': 30}
print(type(g)) # Output: <class 'dict'>
These built-in data types form the foundation of Python programming and are widely used in various applications, from scripting to large-scale software development.