jwt.encode python
jwt.encode python
I'd be happy to help you with that!
jwt.encode()
is a function from the Python library pyjwt
, which is used for encoding and decoding JSON Web Tokens (JWT). A JWT is a compact, URL-safe way of representing claims to be transferred between two parties.
Here's how it works:
First, you need to install thepyjwt
library. You can do this using pip: pip install pyjwt
. Once installed, you can import the necessary modules and functions:
from jwt import encode, decode, algorithms
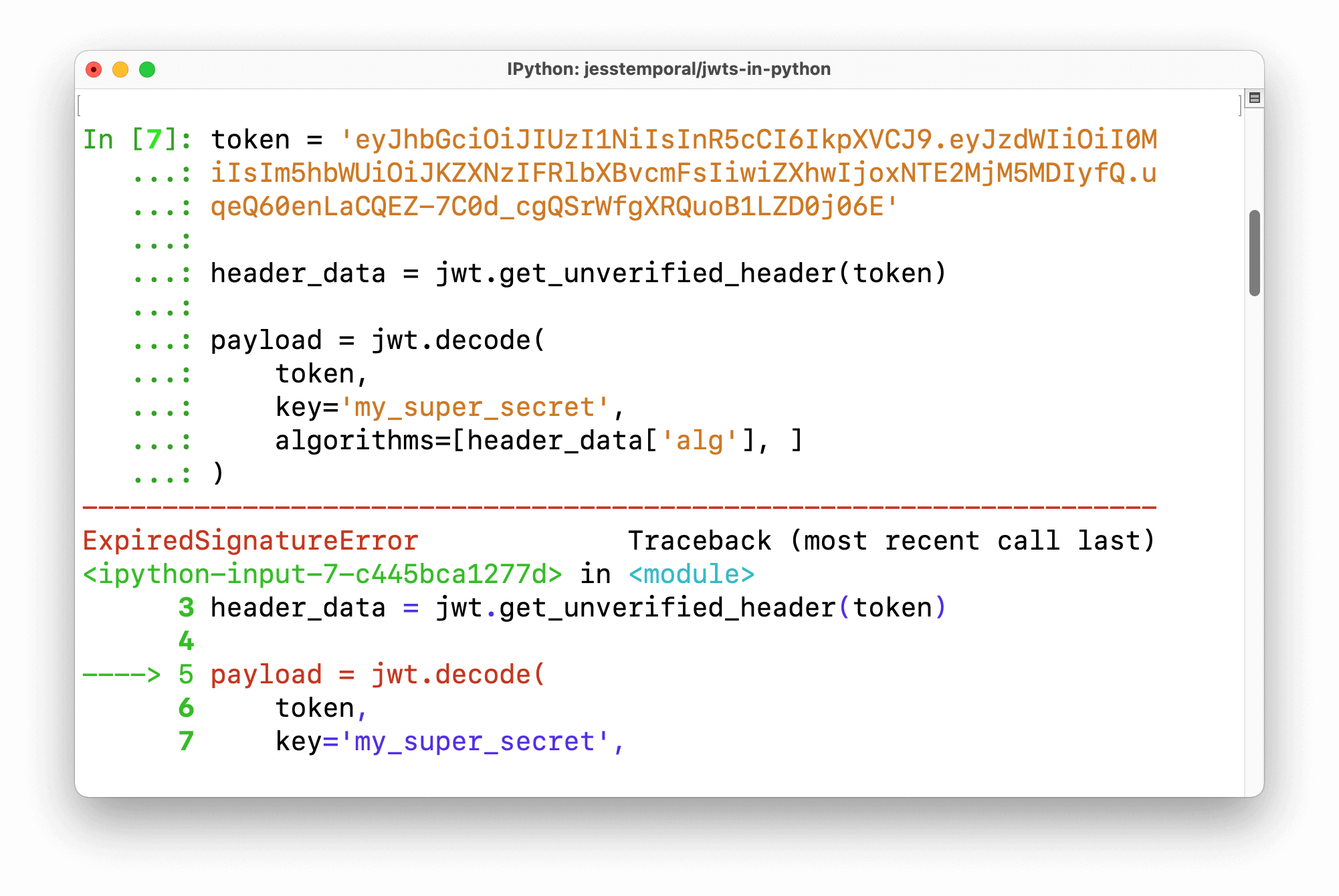
from datetime import datetime, timedelta
Set the algorithm and expiration time for the token
algo = algorithms.HS256 # You can use other algorithms like HS384, RS256, etc.
expires_in = 3600 # One hour
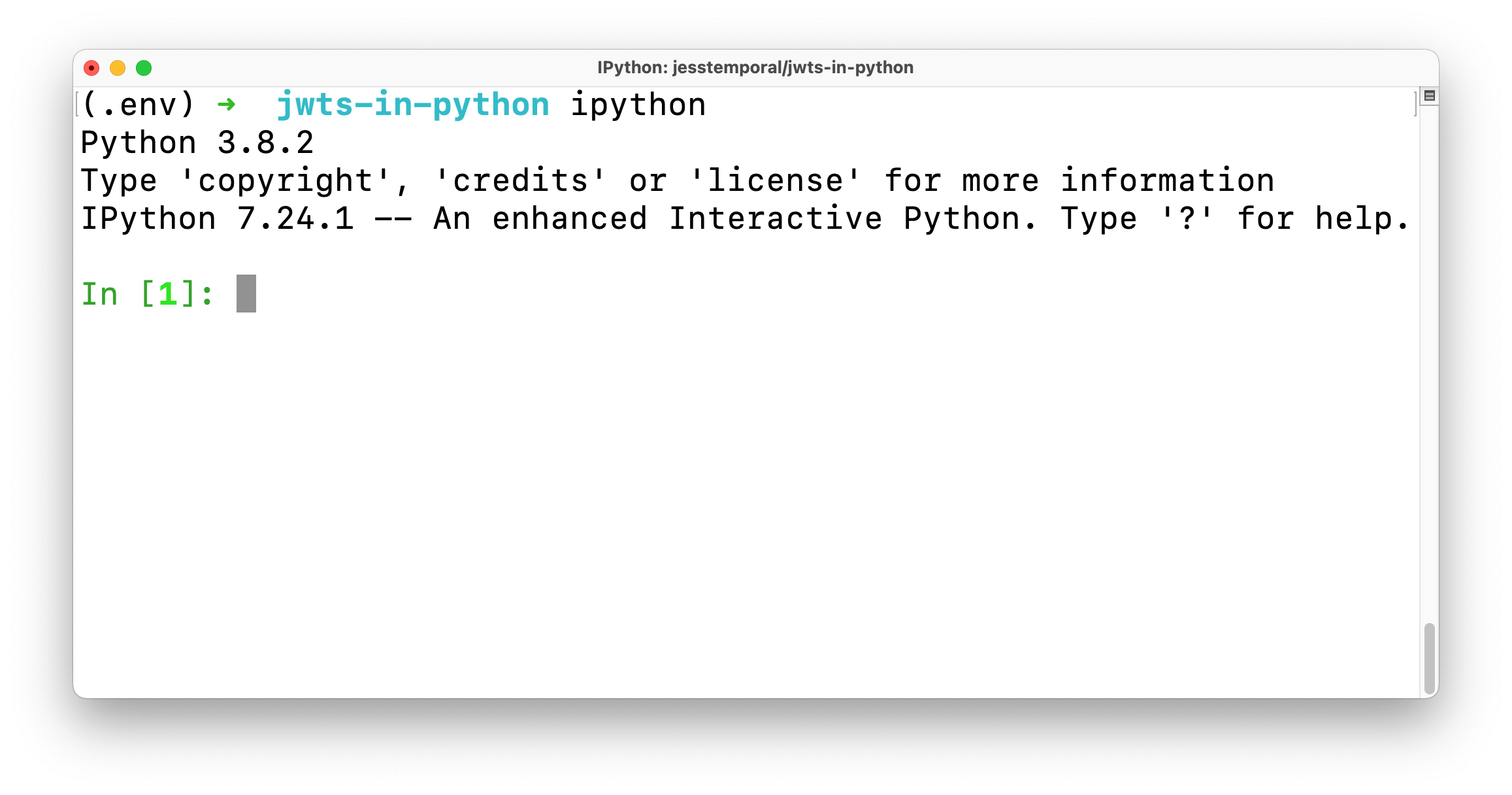
Define a dictionary that contains the claims you want to encode in your JWT:
payload = {
'username': 'your_username',
'email': '[email protected]',
'iat': datetime.utcnow(), # The time at which the token was issued
'exp': datetime.utcnow() + timedelta(hours=1) # The time at which the token expires
}
Use encode()
to create the JWT:
secret = 'your_secret_key' # You can use a secret key or any other secure way to sign your token.
token = encode(payload, secret, algorithm=algo)
Once you have encoded your payload into a JWT, you can verify it by decoding the token and checking if all claims are valid:
def verify(token, secret):
try:
decoded_token = decode(token, secret, algorithms=[algo])
return decoded_token
except Exception as e:
print(f"Invalid token: {e}")
return None
verified_payload = verify(token, secret)
if verified_payload is not None:
print("Token verified successfully!")
Remember to handle exceptions properly and securely store your secret key or any other sensitive information.
Here's the full code:
from jwt import encode, decode, algorithms
from datetime import datetime, timedelta
Set the algorithm and expiration time for the token
algo = algorithms.HS256 # You can use other algorithms like HS384, RS256, etc.
expires_in = 3600 # One hour
Define a dictionary that contains the claims you want to encode in your JWT
payload = {
'username': 'your_username',
'email': '[email protected]',
'iat': datetime.utcnow(), # The time at which the token was issued
'exp': datetime.utcnow() + timedelta(hours=1) # The time at which the token expires
}
Use encode() to create the JWT
secret = 'your_secret_key' # You can use a secret key or any other secure way to sign your token.
token = encode(payload, secret, algorithm=algo)
print(f"JWT Token: {token}")
def verify(token, secret):
try:
decoded_token = decode(token, secret, algorithms=[algo])
return decoded_token
except Exception as e:
print(f"Invalid token: {e}")
return None
verified_payload = verify(token, secret)
if verified_payload is not None:
print("Token verified successfully!")
Hope this helps!
Jwt Python
Here's a comprehensive explanation of JSON Web Tokens (JWT) and how to work with them using Python:
What is JSON Web Token (JWT)?
JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. The tokens are digitally signed and can be verified by anyone in possession of the matching private key.
How does JWT work?
A JWT consists of three parts:
How do you work with JWT in Python?
There are several libraries available for working with JWT in Python. Here are a few popular ones:
PyJWT: This library is based on the PyCryptoDome library and provides a simple way of creating, verifying, and decoding JWTs. jwt-0.3.3: This library provides a more comprehensive set of features for working with JWTs.Here's an example using PyJWT:
from jwt import encode, decode
Define the payload
payload = {"user_id": 123, "username": "John", "roles": ["admin"]}
Set the algorithm and secret key
algorithm = 'HS256'
secret_key = 'your_secret_key_here'
Encode the JWT
jwt_token = encode(payload, secret_key, algorithm=algorithm)
print(jwt_token) # Output: eyJhbGciOiJIUzI1NiIsInR5cCI6I... (shortened for brevity)
Decode the JWT
decoded_payload, _ = decode(jwt_token, secret_key, algorithms=[algorithm])
This example demonstrates how to create a JWT using PyJWT and then verify it.
Common use cases for JWT
Authentication: Use JWTs to authenticate users. You can store the user's ID or username in the payload. Authorization: Store permissions or roles in the payload to control access to specific resources or actions. Session management: Replace traditional session cookies with JWTs, which provide an additional layer of security.Common challenges when working with JWT
Token expiration: Make sure to set a reasonable expiration time for your tokens and implement token refresh mechanisms. Token tampering: Implement checks to prevent tampered tokens from being accepted. Key management: Manage your secret keys securely, as losing access to them can compromise the security of your application.By understanding how JWTs work and using Python libraries like PyJWT or jwt-0.3.3, you can effectively implement secure authentication and authorization mechanisms in your web applications.
Additional resources
JSON Web Token: The official specification for JSON Web Tokens. PyJWT documentation: Learn more about the PyJWT library, including examples and usage guidelines. jwt-0.3.3 documentation: Explore the features and capabilities of this popular JWT library for Python.