Can Python write to a text file?
Can Python write to a text file?
Yes, Python can definitely write to a text file! In fact, it's one of the most common operations you'll do with files.
In Python, there are several ways to write data to a text file, and I'll cover two main methods: using the open()
function and using the with
statement. Both methods have their own advantages and disadvantages, which I'll outline below.
Method 1: Using the open()
function
The most straightforward way to write to a text file is by opening it with the open()
function:
file = open('example.txt', 'w')
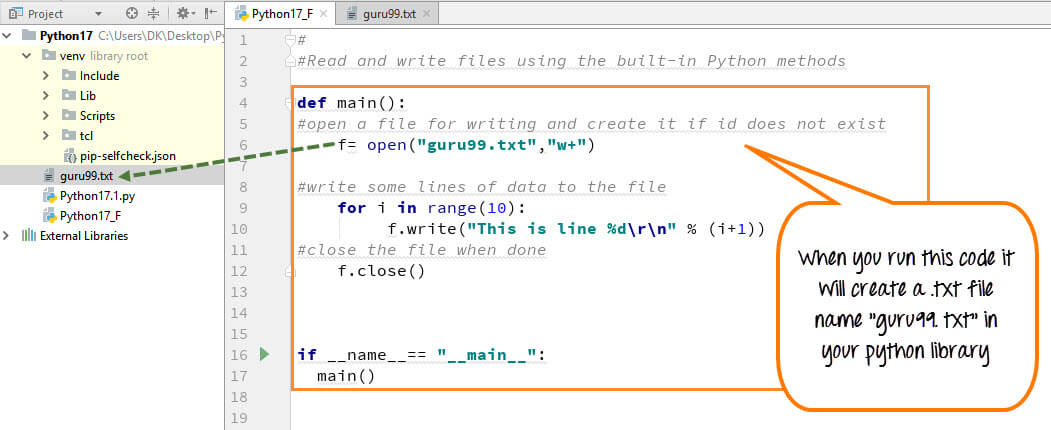
file.write('Hello World!')
file.close()
Here, we specify the filename 'example.txt'
and the mode 'w'
, which stands for "write". This will create a new file if it doesn't exist or overwrite any existing contents.
To write to the file, we use the write()
method and pass in the string 'Hello World!'
. Finally, we close the file using the close()
method. Note that this approach requires explicit closing of the file using file.close()
, which can lead to errors if you forget or accidentally close the file elsewhere in your code.
Method 2: Using the with
statement
A safer and more Pythonic way to write to a text file is by using the with
statement:
with open('example.txt', 'w') as file:
file.write('Hello World!')
Here, we use the with
statement to automatically handle the opening and closing of the file. When we're done writing to the file, Python will take care of closing it for us, even if an exception occurs! This approach is not only more convenient but also safer, as you don't need to worry about forgetting to close the file.
Both methods can be used to write data to a text file. However, I strongly recommend using the with
statement whenever possible, as it's more Pythonic and helps prevent common errors like forgotten file closures.
Now that we've covered how to write to a text file in Python, let me emphasize some important points:
File modes: When opening a file, you need to specify the mode ('r' for read, 'w' for write, or 'a' for append). Make sure you choose the correct mode for your use case. File handling: Remember that files are opened in binary mode by default. If you're working with text files, be sure to open them with the correct encoding (e.g.,'utf-8'
) and specify the newline
parameter if necessary. Error handling: Always handle potential errors when writing to a file. For example, you might want to check if the file was successfully created or written to before proceeding.
By following these guidelines and using one of the two methods I demonstrated, you'll be able to write data to text files with ease in Python!
Python write to text file w3schools
I'd be happy to help!
Writing to a Text File using Python
In this tutorial, we'll learn how to write data to a text file using Python. This is a fundamental operation in programming and will serve as the basis for more complex operations like reading and writing data from various sources.
The open
Function
To write data to a text file, you need to use the open
function, which returns a file object. The syntax for opening a file is:
file = open("filename.txt", "w")
In this case, "filename.txt"
is the name of the file and "w"
stands for "write" mode.
The write
Method
Once you have opened the file using the open
function, you can write data to it using the write
method:
file.write("Hello, world!")
This will write the string "Hello, world!"
to the text file.
Closing the File
After you're done writing to the file, it's a good idea to close the file using the close
method:
file.close()
Example Code
Here's an example code that demonstrates how to write data to a text file:
def write_to_file():
Open the file in write mode
with open("w3schools.txt", "w") as file:
Write some data to the file
file.write("This is W3Schools Python tutorial.n")
file.write("The aim of this tutorial is to learn how to write ")
file.write("data to a text file using Python.")
file.write("n")
file.close()
Call the function
write_to_file()
Output
If you run this code, it will create a new text file called "w3schools.txt"
and write the following data to it:
This is W3Schools Python tutorial.
The aim of this tutorial is to learn how to write data to a text file using Python.
That's it! I hope this helps you understand how to write data to a text file using Python. If you have any questions or need further clarification, please let me know.
Additional Tips
Always use thewith
statement when opening files to ensure that they are properly closed after use. Make sure to specify the correct mode (e.g., "w"
for write mode) and filename. You can also read data from a text file using the read
method. We'll cover this in a future tutorial!
I hope you've learned something new today!