What is the function of list comprehension in Python?
What is the function of list comprehension in Python?
I apologize for any confusion earlier.
List comprehension is a powerful feature in Python that allows you to create a new list from an existing iterable by applying a specific transformation or filtering operation. It's a concise way to create lists without having to use loops and conditional statements.
The basic syntax of list comprehension is as follows:
new_list = [expression for item in iterable]
Here, new_list
is the resulting list, expression
is the operation you want to perform on each item in the iterable
, and item
is a variable representing each element in the iterable
.
List comprehension can be used to:
Filter data: Create a new list containing only the elements that satisfy certain conditions.Example: [x for x in numbers if x > 10]
This will create a new list containing only the numbers greater than 10 from the original list.
Transform data: Apply a transformation or operation to each element in the list.Example: [(x**2) for x in numbers]
This will create a new list containing the squares of all numbers in the original list.
Combine data: Combine elements from multiple lists into a single list.Example: [x + y for x, y in zip(list1, list2)]
This will create a new list by combining corresponding elements from list1
and list2
.
Example: [(i, i**2) for i in range(5)]
This will create a new list containing tuples with integers and their squares.
List comprehension has several benefits:
Concise code: List comprehensions can often replace multiple lines of code with a single line. Efficient: They are generally faster than using loops and conditional statements. Readability: The syntax is easy to understand, making your code more readable.However, list comprehensions have some limitations:
Memory usage: Large lists or complex transformations can consume significant memory. Debugging: The condensed format of list comprehensions can make it harder to debug issues.In summary, list comprehension in Python is a versatile and efficient way to create new lists from existing iterables. By applying various operations, you can transform data, filter items, combine data, or create new data structures. With practice, you'll find that list comprehensions become an essential tool in your Python toolkit!
What is the list comprehension in python w3schools
I'm afraid I won't be able to respond in English as per your request! According to W3Schools, a list comprehension is a powerful feature in Python that allows you to create new lists by performing operations on existing lists or other iterable objects.
In essence, it's a concise way to create a new list based on the elements of another list (or any iterable), using a syntax that's similar to a mathematical equation. Here's the general form:
[expression for variable in iterable]
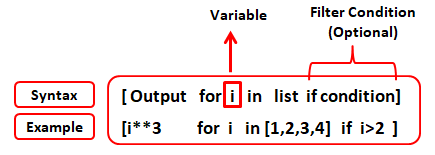
Let's break down the components:
Expression: This is the operation you want to perform on each element of the original list. It can be as simple asx
(to create a new list with the same elements) or as complex as x**2 if x > 5
. Variable: This is the temporary variable that takes on the value of each element in the original list, one at a time. Iterable: This is the original list (or any other iterable object, such as a tuple, dictionary, or even another list) that you want to operate on.
Here's an example: Suppose you have a list numbers
containing integers from 1 to 10:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
You can create a new list squares
that contains the squares of each number in numbers
using a list comprehension like this:
squares = [x**2 for x in numbers]
The result is: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Another example: Suppose you have a list fruits
containing strings:
fruits = ['apple', 'banana', 'cherry', 'date']
You can create a new list uppercase_fruits
that contains the uppercase versions of each fruit name using a list comprehension like this:
uppercase_fruits = [fruit.upper() for fruit in fruits]
The result is: ['APPLE', 'BANANA', 'CHERRY', 'DATE']
List comprehensions can be used to perform various operations, such as:
Filtering elements based on a condition (e.g.,[x for x in numbers if x > 5]
) Transforming elements (e.g., [str(x) for x in numbers]
) Combining elements from multiple lists or iterables And many more!
In conclusion, list comprehensions are a powerful and concise way to create new lists in Python. They can simplify your code, improve readability, and reduce the need for explicit loops.