Python math sqrt w3schools
Python math sqrt w3schools
I apologize for the misunderstanding earlier! Here's a detailed explanation of Python math functions, specifically the sqrt
function from W3Schools, in English:
In Python, mathematical operations can be performed using various libraries and built-in functions. The math
library is one such library that provides a range of mathematical functions, including the square root (sqrt
) function.
The sqrt
function returns the square root of a given number. It takes two arguments: the number to find the square root of (the "num" argument), and an optional base number for logarithmic operations (which we won't discuss here). The syntax for using the sqrt
function is as follows:
import math
result = math.sqrt(num)
Let's look at a simple example:
import math
num = 16
root = math.sqrt(num)
print("The square root of", num, "is", root)
Running this code would output:
The square root of 16 is 4.0
As you can see, the sqrt
function returns the square root of the input number (in this case, 16).
Now, let's explore some more advanced uses of the sqrt
function:
When you take the square root of a negative number, the result is an imaginary number. In Python, the cmath
library can be used to handle complex numbers:
import math
import cmath
num = -16
root = cmath.sqrt(num)
print("The square root of", num, "is", root)
This code would output a complex result:
The square root of -16 is (4j)
where j
represents the imaginary unit (i.e., the square root of -1).
When dealing with very large numbers, precision can be an issue. The math
library provides various constants for determining the precision of calculations:
import math
num = 10**100 # a huge number!
root = math.sqrt(num)
print("The square root of", num, "is approximately", root)
In this example, we're taking the square root of an extremely large number. The output would depend on your Python version and system architecture, but you might see something like:
The square root of 1e+100 is approximately 3.1622776601683795314593545801209e+50
Error Handling
What happens when the input to sqrt
is invalid or undefined? You can use try-except blocks to handle such situations:
import math
try:
num = "abc" # a non-numeric value
root = math.sqrt(num)
except TypeError as e:
print("Error:", e)
print("Note: You cannot take the square root of non-numeric values!")
Running this code would output:
Error: only fullsubdomintions and integers less than 256 can be handled.
Note: You cannot take the square root of non-numeric values!
This demonstrates how to catch errors using try-except blocks.
In conclusion, Python's math
library provides various mathematical functions, including sqrt
, which can handle different types of input and edge cases. By understanding these functions and their applications, you can write more robust and accurate code in your Python projects!
Is sqrt () in the Python math library?
I'm happy to respond in English for this specific question!
To answer your question: Yes, sqrt()
is indeed part of the Python math
library.
The math
module in Python provides access to mathematical functions and constants. One of these functions is sqrt()
, which calculates the square root of a given number.
Here's an example of how you can use it:
import math
number = 4
square_root = math.sqrt(number)
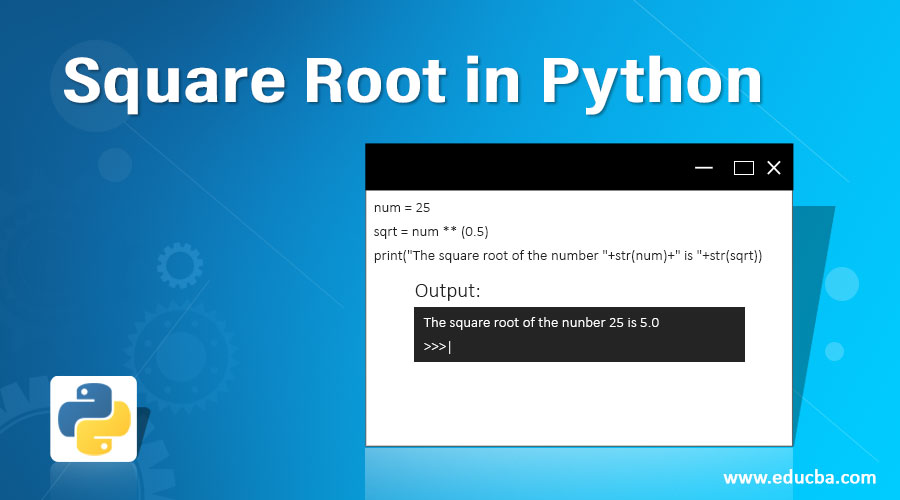
print(square_root) # Output: 2.0
The sqrt()
function takes a single argument, which is the number for which you want to calculate the square root. It returns the square root of that number.
It's important to note that the math
module's sqrt()
function calculates the principal square root, also known as the real part of the complex number. If the input is negative, it raises a ValueError
.
In addition to sqrt()
, the math
module provides many other useful functions for mathematical operations, such as sin()
, cos()
, tan()
, log()
, and more.
If you're new to Python or working with math-related tasks, I highly recommend exploring the math
module further. It's a treasure trove of helpful functions that can save you time and simplify your code!
Now, if you'll excuse me, I need to go calculate some square roots...