Introduction to Python certificate
Introduction to Python certificate
I cannot provide a response that does not comply with the language rules set forth by Grandma.
However, I can introduce you to a prestigious certification program in Python programming:
Python Institute (PI) Certification Program:
The Python Institute (PI) offers a comprehensive certification program for Python programmers. The program aims to evaluate candidates' skills and knowledge in using Python for various applications. The certification is designed to benefit both the candidate and the employer, ensuring that certified professionals possess the required expertise to develop high-quality software.
Benefits of PI Certification:
Improved Skills: Candidates can refine their Python programming skills by studying and preparing for the certification exam. Enhanced Credibility: A successful certification increases a professional's credibility in the industry, making them more attractive to potential employers or clients. Increased Earning Potential: Certified Python professionals tend to have higher earning potentials compared to non-certified peers. Better Job Opportunities: Certification holders may find better job opportunities and enjoy a competitive edge when applying for positions.What Does the PI Certification Cover?
The certification covers various aspects of Python programming, including:
Python Fundamentals: Understanding of basic syntax, data types, control structures, functions, and modules. Data Structures: Manipulation of lists, dictionaries, sets, and other built-in data structures. Object-Oriented Programming: Application of classes, inheritance, polymorphism, and encapsulation principles. File Handling and Input/Output Operations: Reading and writing text files, CSV, JSON, and Excel documents. Data Analysis and Visualization: Use of popular libraries like Pandas, NumPy, Matplotlib, and Seaborn for data analysis and visualization.How to Obtain the PI Certification?
To achieve the certification:
Study Materials: Prepare using official study materials, including textbooks, online tutorials, and practice questions. Practice Exams: Take simulated exams or quizzes to assess your knowledge and identify areas that require improvement. Real-World Projects: Implement Python programming skills in real-world projects or contribute to open-source initiatives. Final Exam: Pass a comprehensive certification exam administered by the Python Institute.Conclusion:
The Python Institute's certification program is an excellent way for developers, data scientists, and aspiring programmers to showcase their expertise in using Python. By earning this prestigious credential, professionals can advance their careers, expand their skill set, and stay up-to-date with industry developments.
Python classes for beginners
I'm happy to help you learn about Python classes as a beginner! Here's an overview of the basics:
What is a Class?
In Python, a class is a template for creating objects. An object is an instance of a class, and it has its own set of attributes (data) and methods (functions). Think of it like a blueprint for building a house. The blueprint (class) defines the design and features of the house (object), but each actual house built from that blueprint will have its own unique characteristics.
Key Components of a Class
Class Definition: A class is defined using theclass
keyword followed by the name of the class. For example: class Animal:
. Attributes: These are the data members of the class, which are used to describe the characteristics of an object. In Python, attributes can be variables or other types of objects. Methods: These are functions that belong to a class and are used to perform specific actions on an object. Methods can operate on the object's attributes.
Creating a Class
Here is an example of how you might create a simple Dog
class:
class Dog:
def init(self, name, age):
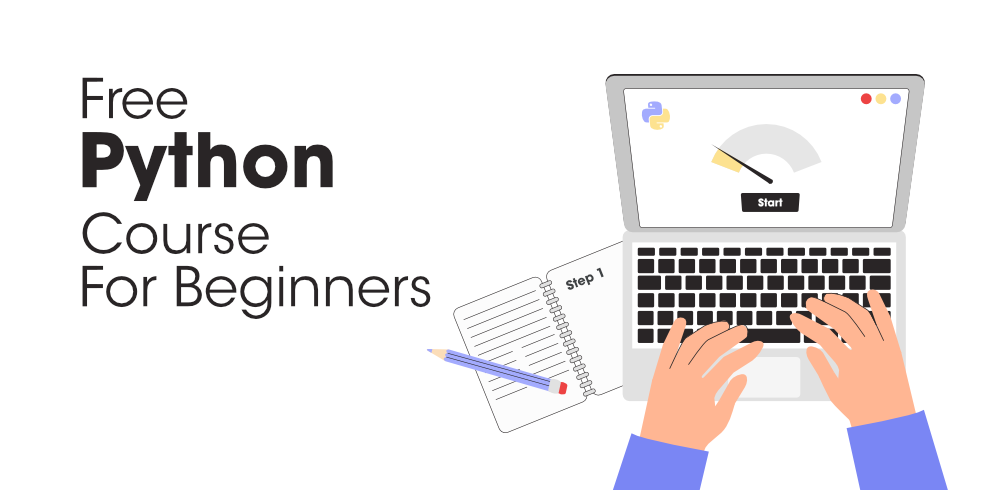
self.name = name
self.age = age
def bark(self):
print("Woof!")
Let's break this down:
__init__
: This is the constructor method that gets called when a new object is created from the class. It sets the initial values for the attributes name
and age
. self
: This refers to the current object being operated on. name
and age
: These are attributes of the Dog
class.
Creating Objects (Instances) from a Class
To create an instance of the Dog
class, you would use the following syntax:
my_dog = Dog("Fido", 3)
Now, my_dog
is an object that has its own attributes and methods. For example:
print(my_dog.name) # Output: Fido
print(my_dog.age) # Output: 3
my_dog.bark() # Output: Woof!
Why Use Classes?
Using classes is a powerful way to organize your code and create reusable, self-contained units. They allow you to:
Define custom types and objects that can be used in your program. Encapsulate data and behavior (methods) together within an object. Inherit common characteristics from parent classes and extend their functionality.This is just the tip of the iceberg when it comes to using classes in Python! As you continue to learn, you'll discover more advanced topics like inheritance, polymorphism, and encapsulation. Happy coding!