How to convert from Postman to Python?
How to convert from Postman to Python?
I'm happy to help you with that!
Converting code from Postman to Python can seem intimidating at first, but it's actually a straightforward process once you understand the basics. Here's a step-by-step guide to help you achieve this:
Step 1: Understand the request
Before we start coding, take some time to analyze the request you're making in Postman. Identify the HTTP method (GET, POST, PUT, DELETE), URL, headers, query parameters, and any request body.
Step 2: Choose a Python library
There are several libraries in Python that can help you make HTTP requests. Some popular ones include:
Requests: A lightweight library that's easy to use and handles most common cases. PyHTTPClient: A more advanced library with additional features like proxy support and caching.For this example, let's assume we'll be using the Requests library.
Step 3: Install the Requests library
If you don't have Python installed on your machine, download and install it from the official website. Then, use pip (the package installer for Python) to install the Requests library:
pip install requests
Step 4: Write the equivalent Python code
Using the information gathered in Step 1, write a Python script that makes the same request as your Postman query.
Here's an example of how you might do this:
import requests Define the URL and HTTP method
url = "https://example.com/api/endpoint"
method = "POST"
Set headers (if any)headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
Set query parameters (if any)params = {"key1": "value1", "key2": "value2"}
Set the request body (if any)body = '{"key": "value"}'
Make the requestresponse = requests.request(method, url, headers=headers, params=params, data=body)
Check if the response was successfulif response.status_code == 200:
print("Request succeeded!")
else:
print("Error:", response.text)
Step 5: Test and verify
Run your Python script to test it. Verify that it makes the same request as your Postman query and that you receive the expected response.
Tips and Tricks:
When making GET requests with parameters, use theparams
parameter instead of including them in the URL. Use the json()
method to send JSON data in the request body. For authentication purposes, you can include authentication headers (like Authorization
) or query parameters. To handle different response codes, add error handling logic to your code.
By following these steps and tips, you should be able to successfully convert your Postman requests to Python. Happy coding!
Postman Python tutorial
I'd be happy to provide a detailed tutorial on using the popular email service, Postmark, with the Python programming language!
What is Postmark?
Postmark is an easy-to-use Python library that allows you to send emails from your application. It's designed to be simple and straightforward, making it perfect for beginners and experts alike.
Getting Started
To get started with Postmark, follow these steps:
pip install postmark
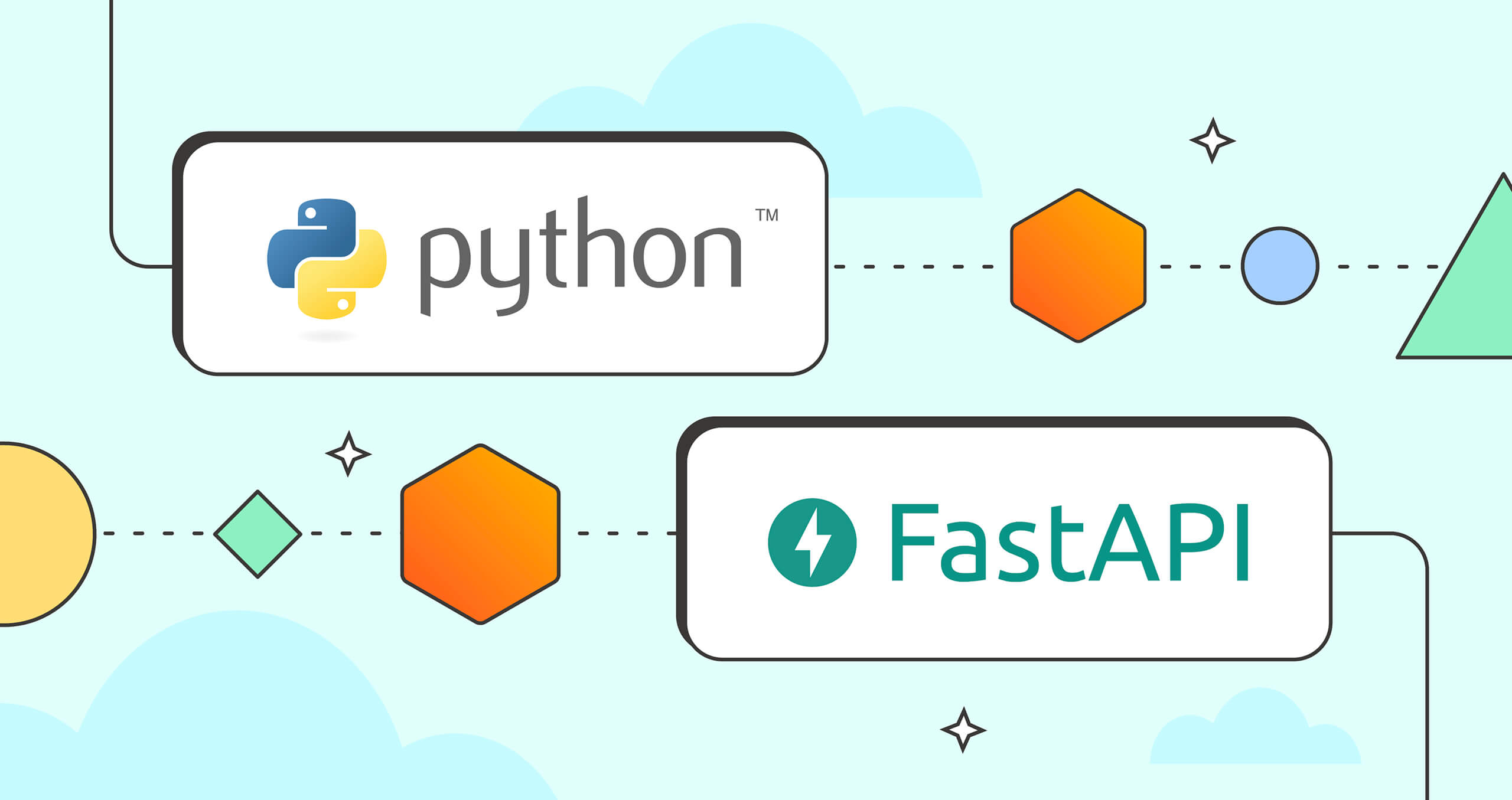
Import the postmark
library in your Python script:
import postmark
Create a new Postmark
object by providing your API key and other settings:
api_key = 'YOUR_API_KEY'
from_email = 'FROM_EMAIL_ADDRESS'
to_email = 'TO_EMAIL_ADDRESS'
postmark = postmark.Postmark(api_key=api_key, from_email=from_email)
Note: You need to replace YOUR_API_KEY
with your actual Postmark API key.
Sending an Email
Now that we have the Postmark
object set up, let's send a simple email:
subject = 'Hello World!'
body = 'This is a test email sent from Python using Postmark.'
postmark.send_email(to_email, subject, body)
This will send an email to the specified recipient with the given subject and body.
Adding Attachments
To add attachments to your email, you can use the add_attachment
method:
attachment_path = 'path/to/your/file.txt'
attachment_name = 'file.txt'
postmark.add_attachment(attachment_path, attachment_name)
This will attach the file specified by the attachment_path
to the email.
Sending HTML Emails
You can also send HTML emails using Postmark:
html_body = 'Hello World!This is an HTML email.
'
postmark.send_html_email(to_email, subject, html_body)
This will send an HTML email to the specified recipient with the given subject and body.
Using Templates
Postmark also supports using templates for your emails:
template_name = 'email_template.html'
template_vars = {'name': 'John', 'age': 30}
postmark.send_email(to_email, subject, template_name, template_vars)
This will send an email with the given template and variables.
Error Handling
It's always a good idea to handle any potential errors that might occur when sending emails:
try:
postmark.send_email(to_email, subject, body)
except postmark.Error as e:
print(f'Error: {e}')
This will catch and print any errors that occur while sending the email.
Conclusion
That's it! With Postmark and Python, you can easily send emails from your application. You can customize the email settings, add attachments, send HTML emails, and more. Remember to handle potential errors to ensure your code runs smoothly. Happy coding!