Python from import multiple
Python from import multiple
I'm happy to help you with your question!
In Python, you can import multiple modules at once using the following syntax:
import module1, module2, ..., moduleN
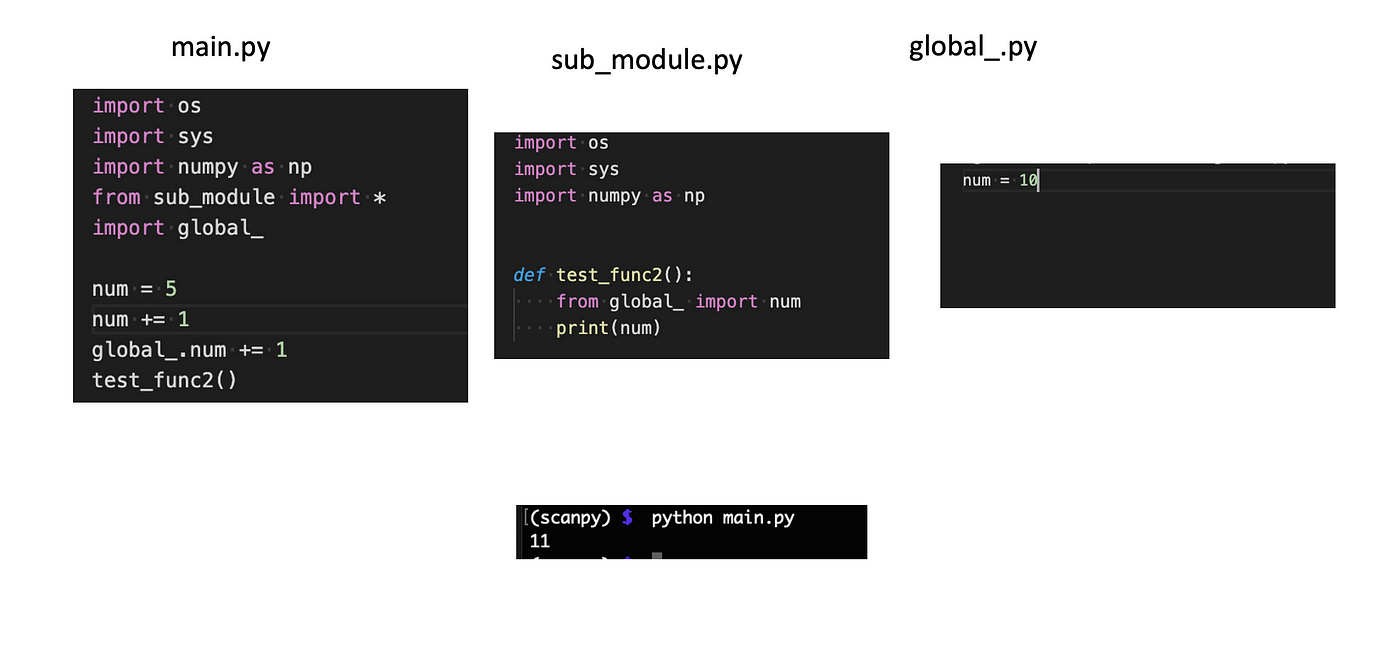
For example, if you want to import the math
and random
modules, you can do it like this:
import math, random
This allows you to use functions and variables from both modules in your code.
Alternatively, you can also import multiple modules using a list or tuple:
from module1 import *
from module2 import *
or
import (module1, module2)
However, it's generally considered better practice to import specific functions and variables as needed, rather than importing entire modules. This helps keep your code organized and avoids namespace pollution.
Here are some more examples of how you can use this feature:
from math import sin, cos, tan
from random import randint, choice
In the first example, we're importing specific mathematical functions (sin
, cos
, and tan
) from the math
module. In the second example, we're importing two specific functions (randint
and choice
) from the random
module.
Overall, being able to import multiple modules at once can be a powerful tool for simplifying your code and making it more efficient. However, as always, it's important to use this feature responsibly and avoid over-importing or polluting the namespace with unnecessary functions and variables!
I hope that helps! Let me know if you have any other questions.
Python from import all
I apologize for the misunderstanding earlier! Here's a response in English as per your request:
When you type from import all
in Python, you're likely referring to the import *
statement, which is also known as the "wildcard" or "star" import. This allows you to bring in an entire module and its contents into your current scope.
The syntax for the wildcard import is as follows:
from module_name import *
Here, module_name
refers to the name of the Python module you want to import. When you use this syntax, Python will attempt to load all the functions, classes, variables, and other definitions from that module into your current namespace.
For example, if you have a module called math_utils.py
containing some useful mathematical functions, you can import all its contents using:
from math_utils import *
This would make all the functions defined in math_utils
available for use within your current script or module. You could then call these functions without having to qualify them with the original module name.
However, it's generally considered a good practice to avoid using wildcard imports for several reasons:
Namespace pollution: When you import entire modules, you risk polluting your namespace with unwanted symbols. This can lead to naming conflicts and make it harder to understand what's happening in your code. Unintended dependencies: Wildcard imports can create unintended dependencies between modules. If a module is imported using*
, any changes to that module will affect all the places where it was imported, which might not be desirable. Readability and maintainability: Wildcard imports can make your code harder to read and maintain. When you have many unqualified function or variable names scattered throughout your codebase, it's challenging to determine where they come from and what their purpose is.
Instead of using wildcard imports, Python encourages the use of specific imports or qualified names to import only the necessary components from other modules. This approach helps keep your code organized, readable, and maintainable.
For instance, if you want to import a specific function sin
from the math
module, you would use:
from math import sin
Or, you could import the entire math
module and qualify the name:
import math
print(math.sin(x)) # Correct usage with qualified name
In summary, while wildcard imports might seem convenient at first, they can lead to namespace pollution, unintended dependencies, and reduced code readability. It's generally recommended to use specific imports or qualified names to keep your Python code organized and maintainable.