Python async file watcher
Python async file watcher
Here's a comprehensive guide on creating an asynchronous file watcher using Python:
Why Asynchronous?
When dealing with file watching, you often want to monitor multiple files simultaneously without blocking the main thread or causing I/O bottlenecks. This is where asynchronous programming comes in handy. By leveraging async/await syntax and libraries like asyncio, you can create non-blocking file watchers that allow your program to continue executing while waiting for file changes.
The Code:
Here's a simple example of an asynchronous file watcher using Python 3.5+:
import asyncio
import os
from watchdog.observers import Observer
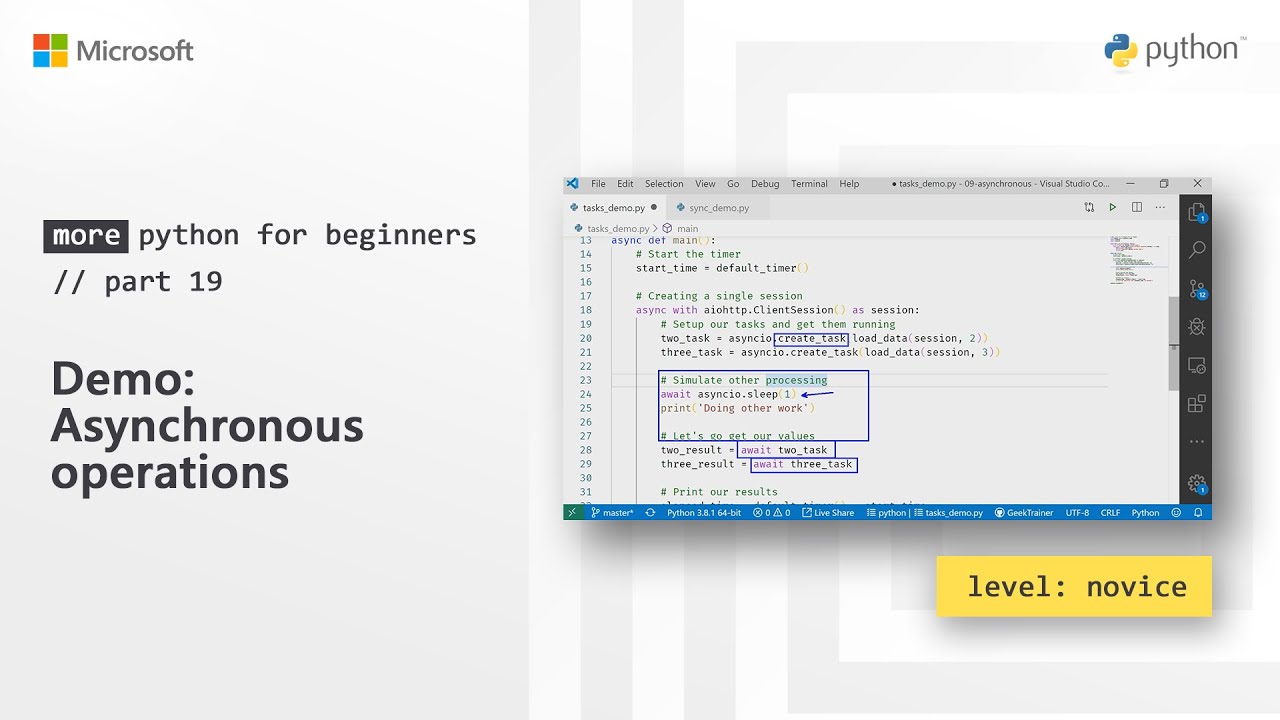
class FileWatcher:
def init(self, directory):
self.directory = directory
self.observer = Observer()
self.watch()
async def watch(self):
self.observer.start(path=self.directory)
while True:
events = await self.observer.event_queue.get_events()
for event in events:
if event.is_directory:
continue # ignore directory changes
print(f"File changed: {event.src_path}")
perform actions based on the file change (e.g., recompile, notify)
await asyncio.sleep(0.1) # avoid overloading the CPU
def stop(self):
self.observer.stop()
self.observer.join()
if name == "main":
watcher = FileWatcher("/path/to/your/directory")
try:
loop = asyncio.get_event_loop()
loop.run_until_complete(watcher.watch())
except KeyboardInterrupt:
watcher.stop()
How it works:
Initialize theFileWatcher
class with a target directory. Start the file observer using Observer.start()
. In the main loop, consume events from the event queue using event_queue.get_events()
(async). Iterate over the received events and handle them accordingly. For this example, we simply print the changed file path. Use await asyncio.sleep(0.1)
to avoid overloading the CPU by consuming too many events in rapid succession.
Advantages:
Non-blocking I/O operations reduce the risk of blocking your main thread or causing performance issues. Asynchronous programming allows your program to continue executing while waiting for file changes, making it more responsive and efficient.Limitations:
This is a basic example and may not cover all edge cases. You should adapt this code to suit your specific needs. Depending on the complexity of your file watching logic, you might need to handle errors or exceptions differently.By leveraging asyncio and async/await syntax, we can create efficient and responsive file watchers that won't block your main thread. This is just a starting point; feel free to modify it as needed for your project!
How to use asyncio in Python?
I'd be happy to explain how to use asyncio
in Python.
What is asyncio?
asyncio
is a Python library that allows you to write single-threaded, concurrent code using coroutines, multiplexing I/O access over sockets and other resources, and implementing network clients and servers. It provides support for both high-level abstractions (e.g., run_coroutine_until_complete
) as well as low-level functionality (e.g., loop
, create_task
).
Why use asyncio?
You might want to use asyncio
if you:
asyncio
can help you achieve this. Want to improve the responsiveness of a blocking I/O-bound application: By using non-blocking coroutines and asynchronous I/O operations, you can avoid the need for threads and still maintain responsiveness in your application. Need to write scalable network servers: asyncio
provides support for writing network servers that are designed to be highly concurrent and scalable.
How to use asyncio?
Here's a basic example of how to use asyncio
:
import asyncio
async def hello_world():
print('Hello')
await asyncio.sleep(1) # wait for 1 second
print('World')
loop = asyncio.get_event_loop()
loop.run_until_complete(hello_world())
This code defines a coroutine named hello_world
, which prints 'Hello', waits for one second using asyncio.sleep
, and then prints 'World'. The run_until_complete
function is used to run the coroutine until it completes.
Here's another example of how to use asyncio
with multiple coroutines:
import asyncio
async def hello():
print('Hello')
async def world():
print('World')
Create a list of tasks
tasks = [hello(), world()]
Run all the tasks concurrently using asyncio.gather
asyncio.run(asyncio.gather(*tasks))
This code defines two coroutines, hello
and world
, each of which prints out a message. A list of tasks is created by calling these coroutines. The asyncio.gather
function is used to run all the tasks concurrently.
Key concepts in asyncio
Here are some key concepts that you should understand when using asyncio
:
Best practices for using asyncio
Here are some best practices to keep in mind when using asyncio
:
await
to pause and resume coroutines as needed. Avoid deep recursion: asyncio can cause recursion errors if you're not careful, so try to avoid using recursive functions.
Common mistakes when using asyncio
Here are some common mistakes to avoid when using asyncio
:
await
correctly, your coroutines may not run concurrently as expected. Blocking in a coroutine: If you perform blocking operations within a coroutine, you can cause the entire event loop to block. Failing to handle exceptions: Make sure to catch and handle exceptions properly in your coroutines.
Overall, asyncio
is a powerful library that allows you to write concurrent code using coroutines, multiplexing I/O access over sockets and other resources, and implementing network clients and servers. By understanding how to use asyncio
correctly and avoiding common mistakes, you can take advantage of its concurrency features and create high-performing Python applications.