How do you write to a file in Python with open?
How do you write to a file in Python with open?
I'd be happy to explain how to write to a file in Python using the open()
function!
In Python, writing to a file is a fundamental operation that involves manipulating text files or binary data files. The open()
function is used to create and open files for reading and/or writing.
Here's an example of how you can use the open()
function to write to a file:
# Define the filename and mode
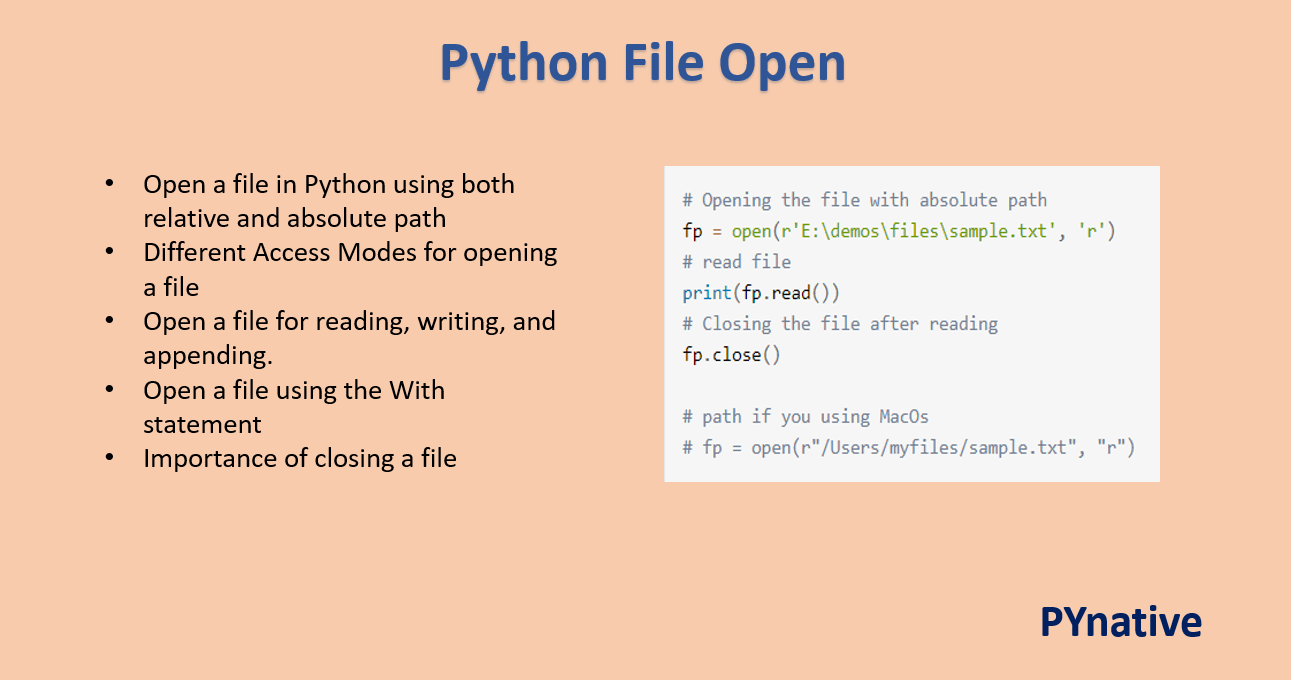
filename = 'example.txt'
mode = 'w' # 'w' stands for "write" - overwrite if it exists
try:
Open the file in write mode
with open(filename, mode) as f:
Write some data to the file
f.write('Hello, world! n')
f.write('This is an example text file. n')
except IOError as e:
print(f"Error writing to {filename}: {e}")
In this code:
We first import theopen()
function from the built-in Python library. We then define a filename and mode for our operation. In this case, we want to write some text data to a file named example.txt
. The mode 'w'
means "write" - if the file already exists, it will be overwritten. Next, we use the open()
function to open the specified file in the defined mode. We then enter a try/except
block, which is used to catch any exceptions that might occur during our operation.
Inside the try
block:
write()
method. If an error occurs while writing to the file (for example, if the file doesn't exist or if you don't have permission to write to it), the except
block will be executed.
Here are a few things to note:
The mode'w'
truncates the file, meaning that if the file already exists, its contents will be deleted. If you want to append to an existing file instead of overwriting it, use the mode 'a'
. You can also specify additional options when opening a file using open()
. For example, you can set the file encoding (e.g., 'utf-8'
) or open the file in binary mode (e.g., 'rb'
for reading or 'wb'
for writing). Finally, it's always a good idea to use the with
statement when working with files. This ensures that the file is properly closed and any system resources are released, even if an error occurs while you're writing to the file.
I hope this explanation helps!
How to write into a text file in Python?
I'd be happy to help! Here's how you can write data into a text file using Python:
Python provides several ways to handle files, including the ability to read and write text files. You can use the built-in open function to create a new file or open an existing one.
Here is a simple example of writing text into a file:
file = open("output.txt", "w")
Write some lines
file.write("This is line 1.n")
file.write("This is line 2.n")
file.write("This is line 3.n")
Close the file when you're done with it
file.close()
In this example, we are creating a new file named "output.txt" and writing three lines of text into it. The "w" in open("output.txt", "w")
means write mode - if the file does not exist, Python will create it; otherwise, it will erase any existing data.
However, there's an even easier way to do this using a context manager with the with
statement:
with open("output.txt", "w") as file:
file.write("This is line 1.n")
file.write("This is line 2.n")
file.write("This is line 3.n")
The with
statement automatically takes care of closing the file for you, so it's a good idea to use this way if you're not sure you'll remember to close the file.
If you want to write data into a text file without specifying the exact lines you want to write, Python also allows you to create a list and then write that list to the file:
with open("output.txt", "w") as file:
my_list = ["This is line 1.", "This is line 2.", "This is line 3."]
for line in my_list:
file.write(line + "n")
In this example, we are creating a list of strings and then writing each string to the file on its own line.
There's one more thing you should know about when working with files - the try/except block. Python will throw an exception if there is a problem reading or writing a file (like if the file doesn't exist, or if it can't be written to because of permissions issues). You should always wrap your file handling code in a try/except block so that your program won't crash if something goes wrong:
try:
with open("output.txt", "w") as file:
my_list = ["This is line 1.", "This is line 2.", "This is line 3."]
for line in my_list:
file.write(line + "n")
except Exception as e:
print(f"An error occurred: {e}")
By using try/except blocks and the with
statement, you can make your program more robust and easier to work with.
Remember that Python's open
function returns a file object that has its own set of methods for reading from and writing to files. The main methods are:
read()
: Read the contents of a text file. write()
: Write some data to a text file. close()
: Close the file when you're done with it.
It's also worth noting that Python has several built-in modules for handling different types of files. The most common one is the os
module, which contains functions for working with the operating system and its files.