Array and linked list in Python
Array and linked list in Python
I'm happy to provide you with information on arrays and linked lists in Python. Here's a detailed explanation:
Arrays in Python:
In Python, an array is not exactly the same as an array in other languages like Java or C++. Instead, it's more similar to a list, which is a data structure that can store multiple values. In Python, you don't need to declare the size of an array before using it; instead, you can create an empty list and keep adding items to it.
Here's how you can create and use arrays in Python:
# Create an empty array (list)
my_array = []
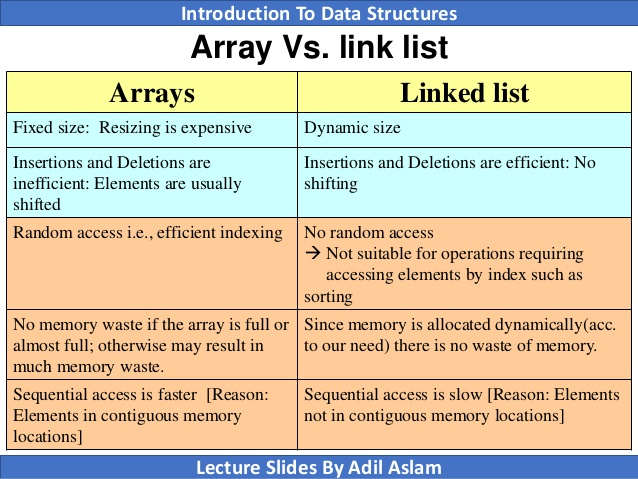
Add elements to the array
my_array.append(1)
my_array.append(2)
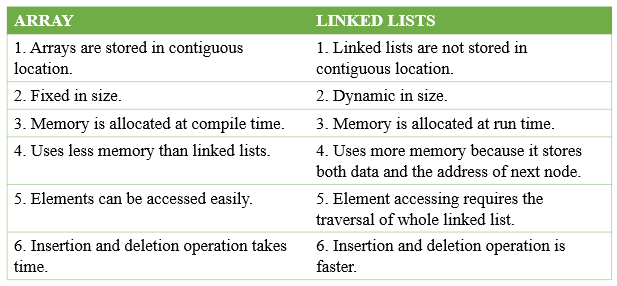
my_array.append(3)
print(my_array) # Output: [1, 2, 3]
Arrays in Python are dynamic and can grow or shrink as needed. You can also use indexing to access specific elements in the array:
# Create an empty array (list)
my_array = []
Add elements to the array
my_array.append(1)
my_array.append(2)
my_array.append(3)
print(my_array[0]) # Output: 1
print(my_array[-1]) # Output: 3
Linked Lists in Python:
A linked list is a data structure where each element, called a node, contains a reference (or "link") to the next node. In Python, you can create a linked list by creating separate objects for each node and linking them together:
class Node:
def init(self, value):
self.value = value
self.next = None
class LinkedList:
def init(self):
self.head = None
def add(self, value):
new_node = Node(value)
if not self.head:
self.head = new_node
else:
current_node = self.head
while current_node.next:
current_node = current_node.next
current_node.next = new_node
def print_list(self):
current_node = self.head
while current_node:
print(current_node.value)
current_node = current_node.next
Create a linked list and add elements to it
my_list = LinkedList()
my_list.add(1)
my_list.add(2)
my_list.add(3)
print("Linked List:")
my_list.print_list() # Output: 1, 2, 3
In this example, each node contains a value and a reference to the next node. The LinkedList class allows you to add nodes (or elements) to the list and print out all the elements.
Key differences between arrays and linked lists in Python:
Memory usage: Arrays use more memory than linked lists because they store all their elements in a contiguous block of memory, whereas linked lists only store pointers to each node. Random access: Arrays allow for random access, meaning you can quickly retrieve any element from the array by its index. Linked lists, on the other hand, require traversing the list to find a specific element. Insertion and deletion: Linked lists make insertion and deletion of elements more efficient because they only need to update the pointers of adjacent nodes. Arrays would require shifting all elements after the inserted or deleted element. Search time: Linked lists have a slower search time compared to arrays, since you need to traverse the list from the beginning to find an element.In summary, if you're working with a small number of elements and need fast random access, arrays might be the better choice. If you're dealing with larger data sets or frequently inserting and deleting elements, linked lists could be the way to go.
How do you link lists together in Python?
Linking lists together is a fundamental operation in Python programming that allows you to combine multiple lists into one unified list. This process is also known as concatenation, merging, or combining lists.
In Python, there are several ways to link lists together, depending on the specific requirements and data structures involved. Here are some common methods:
+
Operator: One of the simplest ways to combine lists is by using the plus (+) operator. This method concatenates two lists into a new list.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
new_list = list1 + list2
print(new_list) # Output: [1, 2, 3, 4, 5, 6]
Using the extend
Method: The extend method is a built-in function in Python that allows you to add all elements from another list (or any iterable) to the end of an existing list.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
extend
Method with a Loop: If you have multiple lists and want to concatenate them all together, you can use a loop that iterates over each list and applies the extend method.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
result_list = []
for lst in (list1, list2, list3):
result_list.extend(lst)
print(result_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Using the chain
Function from the itertools
Module: Another way to concatenate lists is by using the chain function from the itertools module. This method allows you to link multiple lists together in a single step.
from itertools import chain
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
result_list = list(chain(list1, list2, list3))
print(result_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In conclusion, linking lists together in Python involves using various methods such as the +
operator, extend method, loop with extend, and the chain function from itertools. Each method has its own advantages and disadvantages, depending on the specific requirements of your project.
Additional Tips
Make sure to understand the difference between mutable and immutable data types in Python. When working with large datasets, it's essential to optimize your code for performance by using efficient algorithms and data structures. Always test your code thoroughly to ensure that it produces the expected output and handles edge cases correctly.