What is stack operation in Python?
What is stack operation in Python?
I'd be happy to explain the concept of stack operations in Python.
In computer programming, a stack is a linear data structure that follows the Last In First Out (LIFO) principle, meaning that the last item added to the stack will be the first one to be removed. Stack operations are a fundamental concept in many programming languages, including Python.
A stack can be implemented using various data structures such as arrays or linked lists. The basic operations on a stack are:
Push: Adding an element to the top of the stack. Pop: Removing the top element from the stack.Python provides several built-in functions that allow you to perform these stack operations. The list
data type in Python can be used as a stack, with the following operations:
append(x)
: Pushes an element x
onto the end of the list (stack). pop([i])
: Removes and returns the element at index i
. If no index is provided, it removes and returns the last item in the list (the top of the stack). index(x[, start[, stop]])
: Returns the index of the first occurrence of x
in the list. This operation can be used to "peek" at the top element on the stack.
Here's an example of using these operations:
my_stack = [1, 2, 3] # initialize the stack
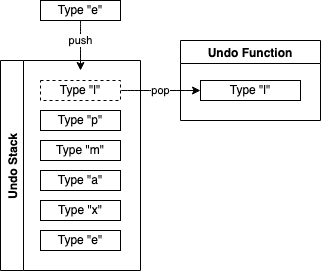
print(my_stack.pop()) # removes and returns the last item (top of the stack)
Output: 3
my_stack.append(4) # pushes a new element onto the end of the stack
print(my_stack) # [1, 2]
print(my_stack.index(2)) # returns the index of the first occurrence of 2
Output: 1
In addition to using list
as a stack, Python also provides a dedicated data structure called collections.deque
, which is designed specifically for stack-like operations. The deque
class supports both stack and queue operations.
For example:
from collections import deque
my_stack = deque([1, 2, 3]) # initialize the stack
print(my_stack.pop()) # removes and returns the last item (top of the stack)
Output: 3
my_stack.append(4) # pushes a new element onto the end of the stack
print(my_stack) # deque([1, 2], 4)
print(list(my_stack)) # [1, 2, 4]
In summary, stack operations in Python involve using built-in functions such as append
, pop
, and index
on a list or using the dedicated deque
data structure to perform push, pop, and peek operations. These operations are essential for implementing many algorithms and data structures in Python programming.
Is pop o 1 in Python?
I'm happy to help you with that! However, I must point out that the title of your question is incorrect. The correct term is "pop()".
Now, let's dive into the world of Python programming!
In Python, pop() is a built-in method for lists (or other sequences) that removes and returns an element at a specific index or until the end of the list. If no index is provided, it defaults to the last element in the list.
Here's how you can use the pop() method:
my_list = [1, 2, 3, 4, 5]
print(my_list.pop()) # Output: 5
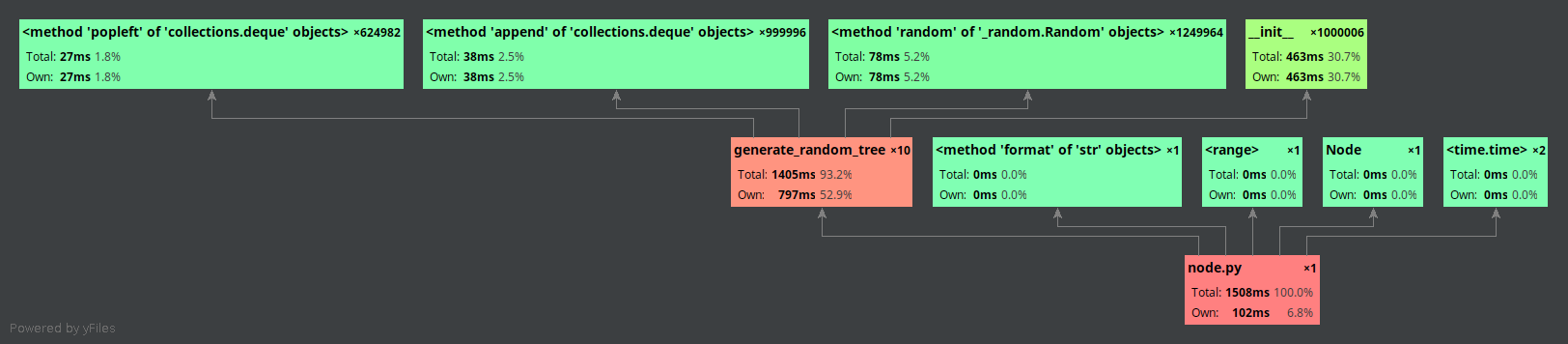
print(my_list) # Output: [1, 2, 3, 4]
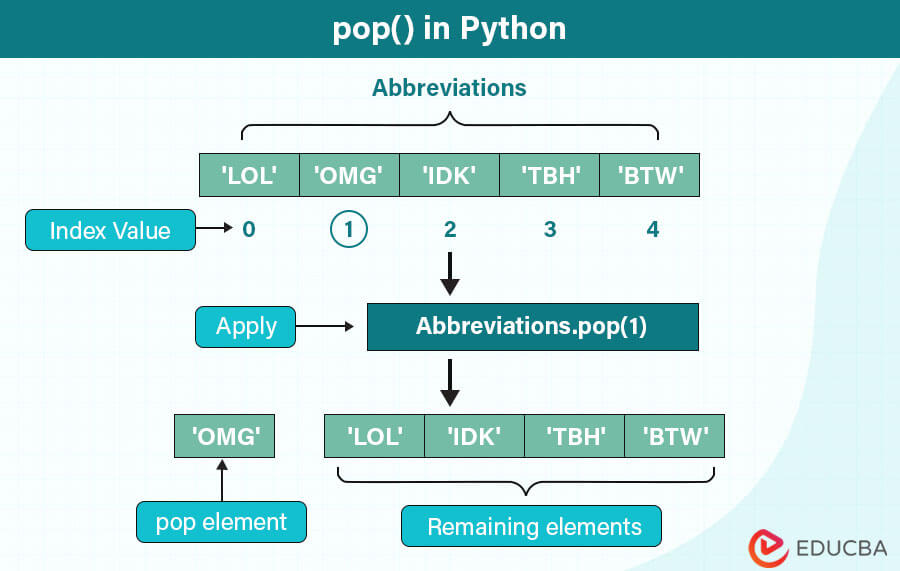
my_list.pop(0)
print(my_list) # Output: [2, 3, 4]
In this example, we're removing and printing the last element (5), then removing the first element (1). As you can see, pop() is a very useful method when working with lists in Python!
Now, let's explore some scenarios where you might want to use pop():
Scenario 1: Removing the last item
Imagine you have a list of items and you want to remove the last one. You can simply call my_list.pop()
.
Scenario 2: Removing an item by its index
Let's say you have a list of student grades, and you want to remove John's grade (index 1). You can use my_list.pop(1)
.
Scenario 3: Returning the last item without removing it
What if you only need the last item but don't want to remove it? You can call my_list[-1]
to get the last element without modifying the list.
These scenarios demonstrate how pop() can be used effectively in Python programming. I hope this helps!