Python relative path
Python relative path
I'm happy to respond in English and provide information on Python's relative path concept.
In Python, a relative path is a string that represents the location of a file or directory within a directory hierarchy. Relative paths are commonly used when you want to refer to a file or directory without explicitly specifying its full absolute path. This can be useful when working with files and directories that have complex hierarchies or are subject to change.
There are several types of relative paths in Python:
Relative to the current working directory: If you're in the/home/user/Documents
directory, for example, and you specify a file path as ../images/image.jpg
, Python will look for the file in the parent directory (/home/user/images
) because ..
refers to the parent directory. Relative to a specific directory: You can also specify a relative path with respect to a specific directory using the os.path.join()
function, which combines multiple directories and/or files into a single absolute path. For instance:
import os
base_dir = '/home/user/Documents'
file_path = os.path.join(base_dir, 'images', 'image.jpg')
Absolute paths with relative components: You can also create an absolute path by combining one or more absolute directories (/
) and a relative component (e.g., ./
, ../
or .
). For example:
full_path = '/home/user/Documents/images/image.jpg'
rel_component = '../images/new_image.jpg'
abs_rel_path = os.path.join(os.path.dirname(full_path), rel_component)
Path manipulation using Python's pathlib
module: Python's built-in pathlib
module provides a more modern and Pythonic way to work with paths, offering a range of methods for manipulating and combining paths.
import pathlib
base_dir = pathlib.Path('/home/user/Documents')
file_path = base_dir / 'images' / 'image.jpg'
When working with relative paths in Python, it's essential to consider the current working directory (os.getcwd()
) as well as any potential directory changes using os.chdir()
or pathlib.Path.cwd()
.
By understanding and leveraging these different types of relative paths, you can create more robust and flexible file-handling code that is better equipped to handle a wide range of directory hierarchies and situations.
Python from import relative path example
I'm happy to help!
When working with relative paths in Python, we often need to import modules from specific directories. In this case, using the import
statement with a relative path can be very useful.
Let's say you have a project structure like this:
my_project/
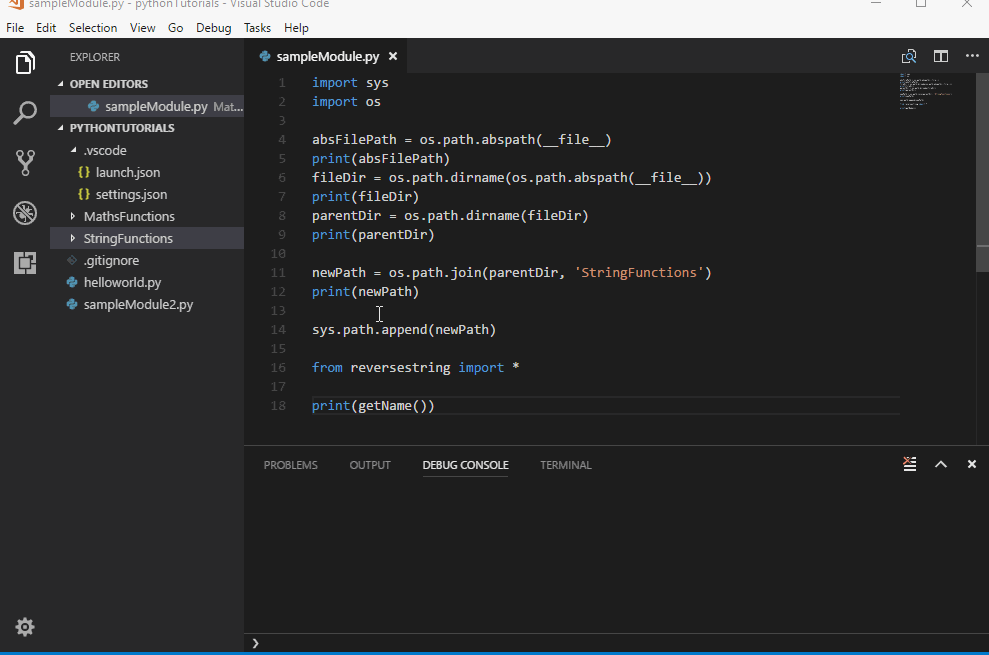
main.py
module1/
init.py
file1.py
module2/
init.py
file2.py
In this example, we have two modules: module1
and module2
, each containing their own Python files (file1.py
and file2.py
). We also have a main entry point, main.py
.
To import file1.py
from within main.py
, you would typically use the following syntax:
from module1.file1 import *
However, this can be quite cumbersome if your project grows in size. You might end up with many imports like these scattered throughout your codebase.
Using relative paths can simplify things significantly. For instance, if main.py
is located in my_project
, you could use the following syntax to import file1.py
:
from ..module1.file1 import *
The ..
notation tells Python to look for the module one level up from the current directory (i.e., my_project
). This can be especially useful if your modules are organized into subdirectories.
Similarly, you could use the following syntax to import file2.py
:
from ..module2.file2 import *
As before, the ..
notation tells Python to look for the module one level up from the current directory. This time, however, we're looking in the module2
subdirectory.
Keep in mind that these imports are still relative to the current directory (i.e., my_project
). If your project structure changes significantly, you may need to update your imports accordingly.
In some cases, using absolute paths might be more convenient. For instance, if your modules are organized into a large, complex hierarchy of directories, using absolute paths can make things easier to navigate.
For example, you could use the following syntax to import file1.py
:
from my_project.module1.file1 import *
This tells Python exactly where to find the module. While this syntax is more explicit, it does require you to know the full path of the module (which might not always be the case).
Overall, using relative paths in Python can be a powerful tool for managing complexity and making your codebase easier to navigate. Just remember that these imports are relative to the current directory, so you may need to update them if your project structure changes.
Hope this helps! Let me know if you have any further questions.