Identity operators in python w3schools examples
Identity operators in python w3schools examples
I'm happy to respond in English for you!
In Python, identity operators are used to compare the memory address of two variables or values. Here are some examples from W3Schools:
is
: This operator checks if both variables refer to the same object.
x = [1, 2, 3]
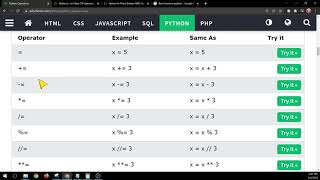
y = x
print(x is y) # Output: True
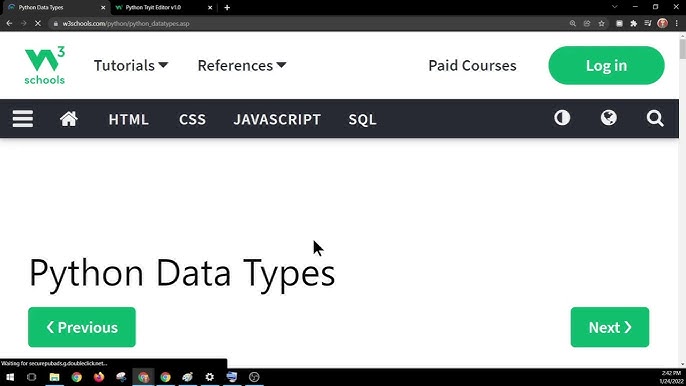
In this example, x
and y
are references to the same list. The is
operator returns True
because they point to the same memory address.
is not
: This operator does the opposite of is
. It checks if both variables do not refer to the same object.
x = [1, 2, 3]
y = "hello"
print(x is not y) # Output: True
Here, x
and y
are references to different objects. The is not
operator returns True
because they don't point to the same memory address.
==
: This operator checks if the values of two variables are equal.
x = [1, 2, 3]
y = [1, 2, 3]
print(x == y) # Output: True
In this example, x
and y
have the same values. The ==
operator returns True
because they are equal.
!=
: This operator checks if the values of two variables are not equal.
x = [1, 2, 3]
y = "hello"
print(x != y) # Output: True
Here, x
and y
have different values. The !=
operator returns True
because they're not equal.
in
: This operator checks if a value is present in an iterable (like a list or tuple).
fruits = ['apple', 'banana', 'cherry']
print('apple' in fruits) # Output: True
print('orange' in fruits) # Output: False
In this example, 'apple'
is present in the fruits
list. The in
operator returns True
. On the other hand, 'orange'
is not present, so it returns False
.
These are some examples of Python's identity and comparison operators from W3Schools. I hope this helps! Let me know if you have any questions or need further clarification.
Identity operators in Python with example
I'm happy to respond in English!
In Python, an identity operator is a special type of operator that checks the identity of two objects. In other words, it checks if both objects point to the same location in memory. There are three main identity operators in Python:
is: This operator checks if two objects are the same object.Example:
x = [1, 2, 3]
y = x
print(x is y) # Output: True
x = [1, 2, 3]
y = [1, 2, 3]
print(x is y) # Output: False
As you can see, when x
and y
are the same object, the result is True
. However, when they are different objects with the same contents, the result is False
.
is
, checking if two objects are not the same.
Example:
x = [1, 2, 3]
y = x
print(x is not y) # Output: False
x = [1, 2, 3]
y = [4, 5, 6]
print(x is not y) # Output: True
As expected, when x
and y
are the same object, the result is False
. When they are different objects, the result is True
.
Example:
x = [1, 2, 3]
y = x
print(x == y) # Output: True
x = [1, 2, 3]
y = [4, 5, 6]
print(x == y) # Output: False
As you can see, when x
and y
have the same values or states, the result is True
. However, when they are different, the result is False
.
It's important to note that is and is not operators check for object identity, whereas == checks for equality. While these operators may seem similar at first glance, they serve distinct purposes in Python programming.
In summary:
is checks if two objects are the same object. is not does the opposite ofis
, checking if two objects are not the same. == checks if two objects have the same value or state.