What is the difference between concurrent and multiprocessing in Python?
What is the difference between concurrent and multiprocessing in Python?
I'll respond in English this time!
The terms "concurrent" and "multiprocessing" are often used interchangeably, but they have distinct meanings in the context of Python programming.
Concurrency
Concurrency refers to the ability of multiple threads or processes to share the same system resources, such as CPU, memory, and I/O devices. In other words, concurrency is about executing multiple tasks simultaneously, without necessarily using multiple cores or processors. This can be achieved through:
Threads: Python has a built-inthreading
module that allows you to create and manage threads within a single process. Coroutines: A coroutine is a special type of thread that can yield control back to the scheduler, allowing other coroutines to run.
Concurrency is useful for tasks that require sharing data or resources between multiple "threads" (e.g., GUI updating, network I/O, and database access). However, concurrency doesn't necessarily take advantage of multi-core processors.
Multiprocessing
Multiprocessing, on the other hand, refers to the use of multiple processes running concurrently, each with its own memory space and potentially utilizing multiple CPU cores. In Python, you can achieve multiprocessing using:
Processes: Themultiprocessing
module allows you to create and manage separate processes, each with its own memory space. Pool: You can use a process pool to execute tasks in parallel, taking advantage of available CPU cores.
Multiprocessing is useful for tasks that:
Can be divided into smaller, independent sub-tasks. Require significant computational resources (e.g., data processing, scientific simulations). Benefit from concurrent execution and the ability to offload work to multiple CPU cores.Key differences between concurrency and multiprocessing in Python:
Concurrency focuses on executing tasks simultaneously within a single process, using threads or coroutines. Multiprocessing involves creating separate processes that can execute concurrently, utilizing multiple CPU cores if available. Concurrency is more suitable for tasks that require sharing resources or data between multiple "threads." Multiprocessing is better suited for tasks that can be divided into smaller, independent sub-tasks and benefit from concurrent execution.In summary:
If you need to perform I/O-bound operations (e.g., network I/O, database access) or share resources between threads, concurrency might be the way to go. If you have computationally intensive tasks that can be parallelized or require significant CPU resources, consider using multiprocessing.Threading vs concurrent futures Python
I'd be happy to help you with that!
Threading and concurrency are fundamental concepts in programming that allow us to execute multiple tasks simultaneously, improving the overall performance and responsiveness of our applications. In this response, I'll explore both approaches using Python as the example programming language.
Threading
In Python, the threading
module provides a way to create and manage threads, which are lightweight processes that can run concurrently with the main program flow. Each thread has its own stack, allowing it to execute separate code without interfering with other threads.
Here's an example of how you can use threading in Python:
import threading
def worker():
print("Worker thread started")
for i in range(5):
print(f"Thread {threading.current_thread().name} is doing some work...")
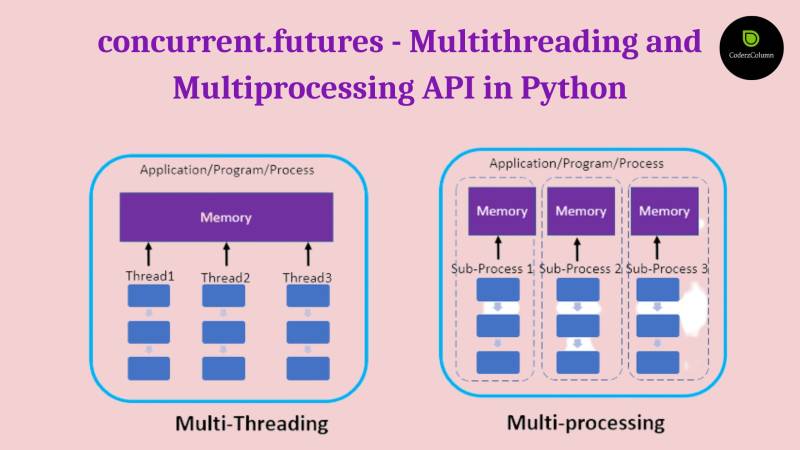
time.sleep(1)
threads = []
for i in range(3):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
Wait for all threads to finish
for t in threads:
t.join()
In this example, we define a worker
function that simulates some work being done. We then create three separate threads that run the worker
function concurrently using the Thread
class. The start()
method starts each thread's execution, and the join()
method ensures that all threads finish before moving on to the next line of code.
Concurrency with concurrent.futures
Python 3.5 introduced a new module called concurrent.futures
, which provides an easier way to write concurrent code using threads or processes. The main advantage of this approach is that you don't need to worry about managing threads and their synchronization manually.
Here's an example of how you can use concurrent.futures
in Python:
import concurrent.futures
def worker():
print("Worker started")
for i in range(5):
print(f"Doing some work...")
time.sleep(1)
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = []
for i in range(3):
future = executor.submit(worker)
futures.append(future)
Wait for all tasks to finish
for future in futures:
future.result()
In this example, we create a ThreadPoolExecutor
instance that manages the execution of our worker function concurrently. We submit each worker function as an independent task using the submit()
method and store the resulting futures in a list. Finally, we use the result()
method to wait for all tasks to finish before moving on.
Comparison
Both threading and concurrency with concurrent.futures
allow you to execute multiple tasks concurrently, but there are some key differences:
concurrent.futures
abstracts away these complexities, making it easier to write concurrent code. Synchronization: When using threading, you typically need to use synchronization primitives (e.g., locks, semaphores) to ensure thread safety. concurrent.futures
uses higher-level abstractions and provides built-in support for concurrency, reducing the need for explicit synchronization. Performance: Threading can be more lightweight than process-based concurrency, as threads share the same memory space. However, Python's Global Interpreter Lock (GIL) means that true parallelism is only achieved when using multiple processes.
In conclusion, both threading and concurrent.futures
are viable options for writing concurrent code in Python. The choice between them depends on your specific use case and requirements. If you need fine-grained control over threads or want to achieve true parallelism, threading might be a better fit. If you prefer a higher-level abstraction that simplifies thread management and concurrency, concurrent.futures
could be the way to go.
I hope this helps! Let me know if you have any further questions.