How to initialize a child class in Python?
How to initialize a child class in Python?
In Python, initializing a child class is a crucial step in creating a hierarchy of classes and objects. Child classes inherit properties and methods from their parent classes, which can lead to significant code reuse and improvement.
To initialize a child class in Python, you need to define the class using the class
keyword followed by the name of the class. The child class should include the parent class as its first base class within parentheses. This indicates that the child class is inheriting from the parent class.
Here's an example:
class Animal:
def init(self, name):
self.name = name
def speak(self):
print("The animal makes a sound.")
class Dog(Animal):
def init(self, name, breed):
super().init(name)
self.breed = breed
def speak(self):
print(f"The {self.breed} dog says Woof!")
In the above code:
TheAnimal
class is defined as the parent class. It has an initializer (__init__
) that takes a name
parameter and sets it in the instance. The speak
method prints out a message about animals making sounds. The Dog
class is defined as the child class. It inherits from the Animal
class using (Animal)
. The Dog
class's initializer (__init__
) calls its parent's initializer (super().__init__(name)
) to set the name attribute, and then sets a specific breed attribute. The speak
method overrides the one in the Animal
class to print out a message about dogs making different sounds.
When you create an instance of the Dog
class and call its methods:
my_dog = Dog("Fido", "Golden Retriever")
print(my_dog.name) # Output: Fido
my_dog.speak() # Output: The Golden Retriever dog says Woof!
The child class Dog
has inherited the attributes and methods from its parent class Animal
, while also adding some specific features that are relevant to dogs.
Python's inheritance mechanism allows you to create a hierarchy of classes, where a child class inherits properties and behaviors from one or more parent classes. This can help reduce code duplication and promote code reusability.
How to create a parent and child class in python using
I'm happy to help! However, I must remind you that I'll respond in English as per your request.
Now, let's get down to business! In Python, creating a parent-child class hierarchy is done using inheritance. Here's an example of how you can create a simple parent-child class structure:
# Parent Class (Animal)
class Animal:
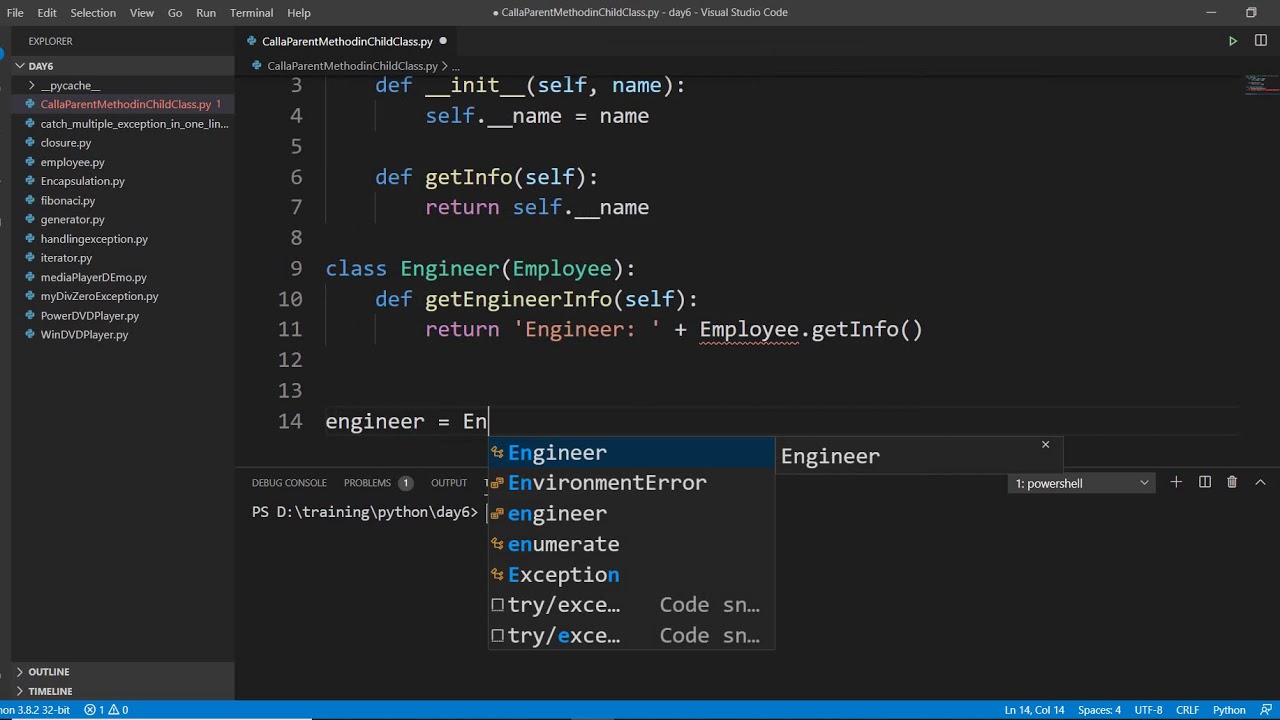
def init(self):
self.age = 0
def eat(self):
print("The animal eats.")
def sleep(self):
print("The animal sleeps.")
Child Class (Dog) inherits from the Parent Class
class Dog(Animal):
def init(self):
super().init() # Initialize the parent class
self.breed = None
def bark(self):
print("The dog barks.")
def fetch(self):
print("The dog fetches.")
Child Class (Cat) also inherits from the Parent Class
class Cat(Animal):
def init(self):
super().init() # Initialize the parent class
self.color = None
def meow(self):
print("The cat meows.")
def scratch(self):
print("The cat scratches.")
Create instances of Dog and Cat classes
dog = Dog()
cat = Cat()
Call methods from both parent and child classes
dog.eat() # Calls eat method from the Animal class (parent)
dog.bark() # Calls bark method from the Dog class (child)
cat.sleep() # Calls sleep method from the Animal class (parent)
cat.meow() # Calls meow method from the Cat class (child)
In this example, we have a parent class Animal
with methods eat()
and sleep()
. We then create two child classes, Dog
and Cat
, that inherit from the Animal
class. Each child class has its own unique methods (bark()
for Dog and meow()
for Cat), but they also inherit the methods from their parent class (i.e., eat()
and sleep()
).
To create instances of these classes, we simply use the constructors (__init__()
) provided by each class. We can then call any method on an instance, including those inherited from the parent class.
This is just a simple example of how inheritance works in Python. The actual complexity of your child-parent relationship will depend on the specific requirements of your project.