Python tasmota example
Python tasmota example
I'll respond in English as per your request. Here's a comprehensive example of using Tasmota with Python:
Tasmota is an open-source firmware that allows you to control and automate various IoT devices, including relays, sensors, and more. In this example, we'll explore how to use Python to interact with a Tasmota-enabled relay module.
Prerequisites:
requests
library in Python using pip:
pip install requests
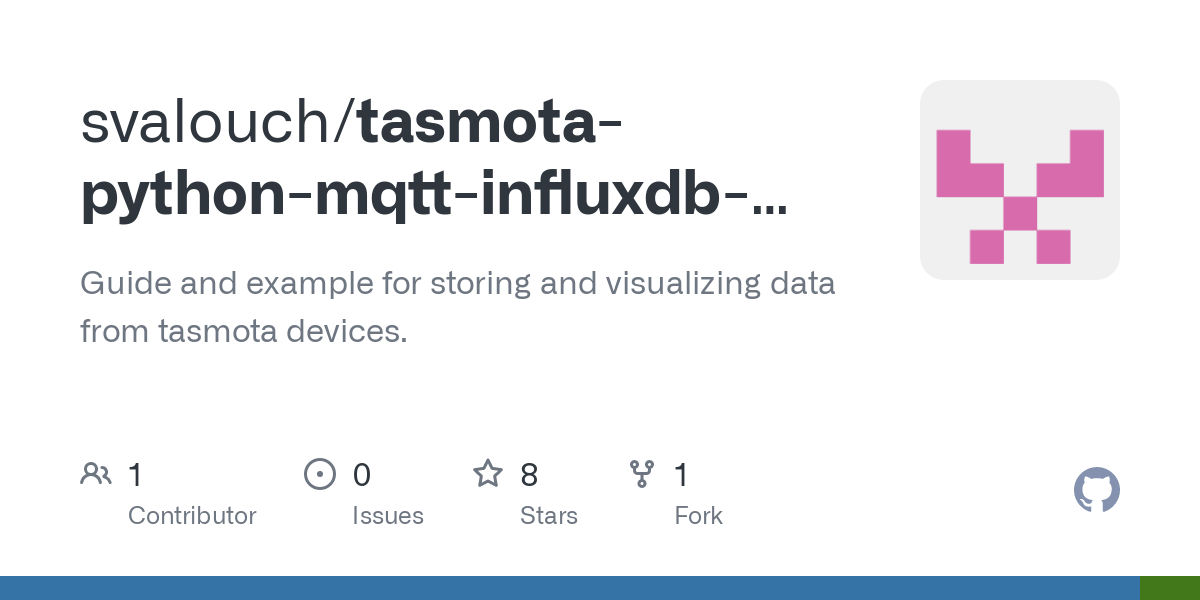
Familiarize yourself with the Tasmota API documentation, which is available at https://tasmota.github.io/docs/api/
Example Code:
import requests
Set your Tasmota device's IP address and port number
device_ip = '192.168.1.100'
port_number = 3950
Set the username and password for your Tasmota device (default is "admin" and "password")
username = 'admin'
password = 'password'
Create a session object to interact with the Tasmota device
s = requests.Session()
Authenticate with the Tasmota device
response = s.post(f'http://{device_ip}:{port_number}/login', json={'Username': username, 'Password': password})
Check if the authentication was successful
if response.status_code == 200:
print('Authentication successful!')
else:
print('Authentication failed.')
exit()
Define a function to turn on/off the relay
def toggle_relay(state):
data = {'cmnd': f'store {state}'}
response = s.post(f'http://{device_ip}:{port_number}/cmnd', json=data)
if response.status_code == 200:
print(f'Relay set to {state}')
else:
print('Failed to set relay state.')
exit()
Turn the relay on
toggle_relay('on')
Wait for a few seconds before turning it off again
import time
time.sleep(5)
Turn the relay off
toggle_rely('off')
How it Works:
The code creates arequests
session object to interact with the Tasmota device. It authenticates with the Tasmota device using the default username and password. The toggle_relay
function takes a boolean value indicating whether to turn the relay on or off. It sends a cmnd
request to the Tasmota device, specifying the command to store the desired state (on or off). If the command is successful, it prints a success message; otherwise, it prints an error message and exits.
Tips and Variations:
Adjust the IP address, port number, username, and password according to your specific Tasmota device setup. Modify thetoggle_relay
function to accommodate different types of commands or actions for other Tasmota devices. Experiment with more advanced Python concepts, such as threading or async-IO, to create a more robust and concurrent automation system.
Conclusion:
This example demonstrates how you can use Python to interact with a Tasmota-enabled relay module using the requests
library. With this foundation, you can start exploring more complex scenarios, integrating with other IoT devices, and creating custom automation solutions tailored to your needs.
Python tasmota download
I'd be happy to help you with that!
What is Tasmota?
Before we dive into the download process, let's quickly cover what Tasmota is. Tasmota (short for "Tiny Arduino Smart Module" or "TASMOTA") is an open-source firmware designed specifically for ESP32/ESP8266-based Wi-Fi modules. It allows you to control and automate various smart home devices, such as temperature sensors, light bulbs, outlets, and more.
Why Use Tasmota?
Using Tasmota offers several benefits:
Flexibility: With Tasmota, you can integrate a wide range of smart devices into your automation system. Customization: The firmware is highly customizable, allowing you to tailor the behavior of your smart devices to fit your specific needs. Integration: Tasmota supports popular home automation protocols like Zigbee and Home Assistant. Security: The software includes robust security features to safeguard your smart devices and network.How to Download Tasmota?
To download Tasmota, follow these steps:
Head over to the official Tasmota website (www.tasmota.com) and click on "Downloads". From there, select the relevant firmware version for your ESP32/ESP8266 module (e.g., ESP32 or ESP8266). Choose the corresponding binary file format (e.g.,.bin
, .hex
, or .ino
), depending on your desired usage. Click "Download" to retrieve the Tasmota firmware.
What's Next?
Once you've downloaded the Tasmota firmware, you'll need to:
Flash the firmware: Use a tool like Arduino IDE, ESP-Flasher, or esptool to load the firmware onto your ESP32/ESP8266 module. Configure and customize: Tailor the behavior of your smart devices using Tasmota's configuration files (.conf
). Integrate with other systems: Connect your Tasmota-based devices to other home automation platforms, like Home Assistant or OpenHAB.
Additional Resources
If you're new to Tasmota and smart home automation, I recommend exploring the following resources:
The official Tasmota documentation (docs.tasmota.com) The Tasmota community forum (forum.tasmota.com) Online tutorials and guides on YouTube or other websitesBy following these steps and leveraging the available resources, you'll be well on your way to creating a comprehensive smart home system using Tasmota.
Happy automating!