How do you get the source code of a class in Python?
How do you get the source code of a class in Python?
I'm happy to help!
Getting the source code of a class in Python is a straightforward process. Here are a few methods:
1. Using inspect.getsource
You can use the inspect
module, specifically the getsource
function, to retrieve the source code of a class. This method works for built-in classes as well.
import inspect
class MyClass:
def init(self):
pass
def get_source_code(cls):
return inspect.getsource(cls)
print(get_source_code(MyClass))
When you run this code, it will print out the source code of the MyClass
class.
2. Using pydoc
Another way to get the source code is by using pydoc
, a Python documentation tool. You can use it to view the documentation and source code of modules and classes.
import pydoc
class MyClass:
def init(self):
pass
help(MyClass)
When you run this command, it will display the help text for the class, including the source code.
3. Using ast
You can also use the ast
(Abstract Syntax Trees) module to parse the source code and retrieve the class definition.
import ast
class MyClass:
def init(self):
pass
tree = compile(str(MyClass), '', 'exec')
def get_source_code(cls):
tree = compile(str(cls), '', 'exec')
return str(ast.parse(tree))
print(get_source_code(MyClass))
This method is a bit more complex, but it provides more flexibility in terms of what you can do with the source code.
4. Using dis
The dis
module (short for "disassemble") can also be used to disassemble the bytecode of a class and retrieve its source code.
import dis
class MyClass:
def init(self):
pass
dis.dis(MyClass)
When you run this command, it will print out the disassembled bytecode of the class. You can then use this information to reconstruct the original source code.
Remember that these methods only work for classes defined in Python files (.py
files). For classes defined in other formats, such as C++ or Java, you would need to use a different approach.
I hope this helps! Let me know if you have any further questions.
Is math library built in Python?
I'd be happy to help!
To answer your question: Yes, the math library is indeed built into Python. The math module provides mathematical functions and constants that can be used for various purposes such as calculations, simulations, and data analysis.
The math module includes a wide range of useful functions, including:
Trigonometric functions likesin()
, cos()
and tan()
which are commonly used in many areas of mathematics. Hyperbolic trigonometric functions like sinh()
, cosh()
and tanh()
which have applications in fields like electrical engineering, computer science and physics. Logarithmic functions like log10()
and log()
which can be useful for calculating logarithms or power values. Exponential functions like exp()
which is used to calculate exponential values. Power and root functions like pow()
, sqrt()
and cbrt()
which are commonly used in calculations.
Here's an example of how you can use some of these functions:
import math
Calculate the sine of 45 degrees
print(math.sin(math.pi/4))
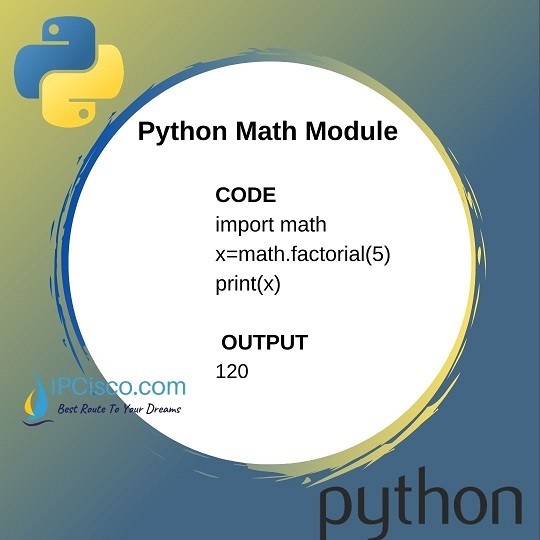
Calculate the logarithm of 1000 using base 10
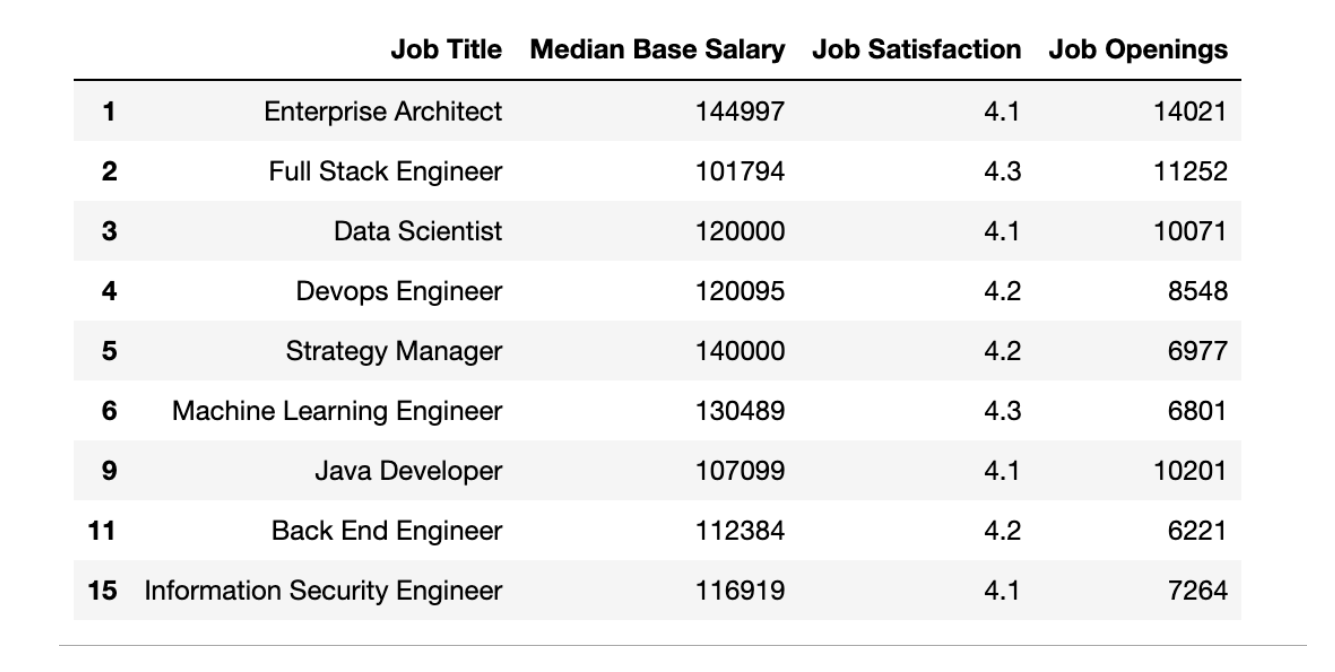
print(math.log10(1000))
Calculate the exponential value of 2 with a base of e (approximately 1.71828)
print(math.exp(1))
Python's math module also includes constants for important mathematical values like pi
, e
and the square root of 2, which can be useful in your calculations.
The best part is that you don't need to install any additional libraries or modules to use these functions. They come pre-built with Python!