How do i authenticate api key in python using
How do i authenticate api key in python using
I'll respond in English for this one.
Authenticating an API key in Python typically involves passing the key as a parameter or header in your HTTP requests. The exact method may vary depending on the API and its authentication requirements, but here are some common approaches:
Parameter-based authentication: In this approach, you pass the API key as a query string parameter when making the request. For example:import requests
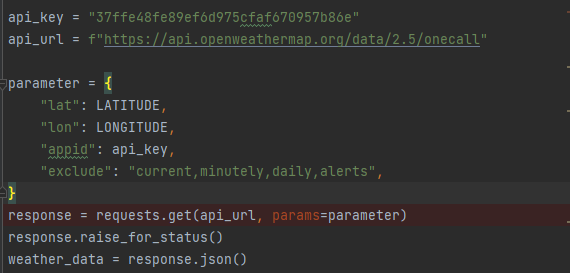
api_key = "your_api_key_here"
url = f"https://api.example.com/endpoint?api_key={api_key}"
response = requests.get(url)
print(response.json())
In this example, we're passing the api_key
as a query string parameter in the URL.
headers
parameter when making the request:
import requests
api_key = "your_api_key_here"
url = "https://api.example.com/endpoint"
headers = {"Authorization": f"Bearer {api_key}"}
response = requests.get(url, headers=headers)
print(response.json())
In this example, we're passing the api_key
as an Authorization
header with a Bearer
scheme. The API will then verify the presence and validity of the key.
import requests
api_key = "your_api_key_here"
url = "https://api.example.com/token"
response = requests.post(url, json={"api_key": api_key})
token = response.json()["token"]
if response.status_code == 200 and token:
Use the token in subsequent requests
headers = {"Authorization": f"Bearer {token}"}
url = "https://api.example.com/endpoint"
response = requests.get(url, headers=headers)
print(response.json())
In this example, we're obtaining an auth token by making a POST request to the /token
endpoint with our API key. We then use that token in subsequent requests as the Authorization
header.
When implementing API key authentication in Python, remember to handle errors and exceptions properly. For example:
try:
response = requests.get(url, headers=headers)
if response.status_code == 401: # Unauthorized
print("Invalid API key")
elif response.status_code == 403: # Forbidden
print("API key not found or invalid")
except Exception as e:
print(f"Error: {e}")
These are some common ways to authenticate an API key in Python. Make sure to consult the API documentation for specific instructions on how to authenticate with their service.
Python requests Authorization header API key
Here's the information you need to know about Python requests and Authorization headers, specifically how to use API keys:
When sending a request using Python's requests
library, it's often necessary to add an Authorization
header that includes an API key or token. This is typically done for security purposes, such as authenticating with a server or accessing premium data.
To start, let's cover the basic structure of an HTTP request in Python using requests
. A simple GET request looks like this:
import requests
response = requests.get('https://api.example.com/data')
However, when working with APIs that require authentication, you'll need to include the API key or token in the Authorization
header. Here's how to do it:
import requests
api_key = 'your_api_key_here'
headers = {'Authorization': f'Bearer {api_key}'}
response = requests.get('https://api.example.com/data', headers=headers)
In this example, replace 'your_api_key_here'
with your actual API key. The f-string
syntax is used to include the API key in the Authorization
header.
import requests
api_token = 'your_api_token_here'
headers = {'Authorization': f'Bearer {api_token}'}
response = requests.get('https://api.example.com/data', headers=headers)
Again, replace 'your_api_token_here'
with your actual API token.
Authorization
header. These tokens are usually generated after authentication and have a specific format:
import requests
auth_token = 'your_auth_token_here'
headers = {'Authorization': f'Bearer {auth_token}'}
response = requests.get('https://api.example.com/data', headers=headers)
Replace 'your_auth_token_here'
with your actual authentication token.
Remember to replace the placeholders (e.g., your_api_key_here
) with your actual API key, token, or authentication credentials. This way, you'll ensure a successful request and avoid any errors due to incorrect or missing authorization information.
When you're finished, you can check the response status code to verify that the request was successful:
print(response.status_code)
If everything went as planned, this should print 200
, indicating a successful request.