Python Selenium Tutorial
Python Selenium Tutorial
I'm happy to help! Here's a comprehensive guide on using Selenium with Python for web scraping and automation.
What is Selenium?
Selenium is an open-source tool used for automating web browsers. It supports multiple programming languages, including Python, Java, C#, Ruby, and more. With Selenium, you can write scripts that interact with websites as if a human was interacting with them.
Python Installation
To use Selenium in Python, you need to install the selenium
library. You can do this using pip:
pip install selenium
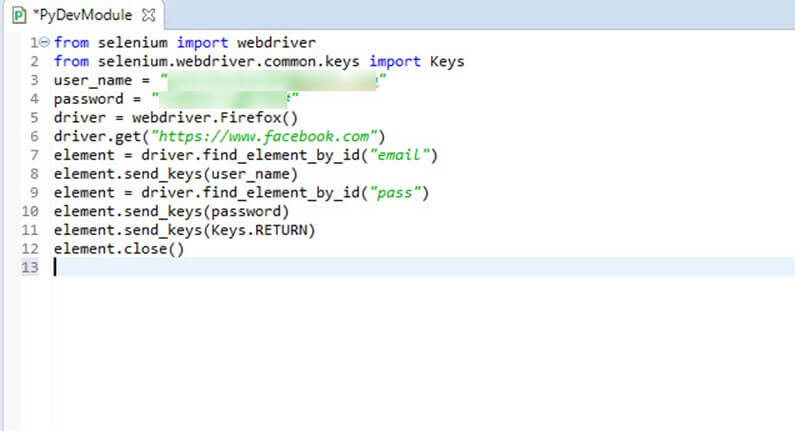
Basic Usage
Here's a simple example of how to use Selenium with Python:
from selenium import webdriver Create a new instance of the Chrome driver
driver = webdriver.Chrome()
Navigate to Google.comdriver.get("https://www.google.com")
Search for "Selenium Tutorial"search_box = driver.find_element_by_name("q")
search_box.send_keys("Selenium Tutorial")
search_box.submit()
Close the browser windowdriver.quit()
In this example, we create a new instance of the Chrome driver using webdriver.Chrome()
. We then navigate to Google.com and search for "Selenium Tutorial". Finally, we close the browser window using driver.quit()
.
Web Scraping
Selenium is particularly useful for web scraping. Here's an example of how you can use Selenium to scrape a website:
from selenium import webdriver
from bs4 import BeautifulSoup
Create a new instance of the Chrome driverdriver = webdriver.Chrome()
Navigate to the websitedriver.get("https://www.example.com")
Parse the HTML content using BeautifulSouphtml_content = driver.page_source
soup = BeautifulSoup(html_content, "html.parser")
Extract the relevant data from the pagedata = []
for element in soup.find_all("div", {"class": "data"}):
data.append(element.text)
Close the browser windowdriver.quit()
print(data) # Print the scraped data
In this example, we create a new instance of the Chrome driver and navigate to a website. We then use BeautifulSoup to parse the HTML content of the page. Finally, we extract the relevant data from the page and print it.
Automating Web Forms
Selenium is also useful for automating web forms. Here's an example of how you can use Selenium to fill out a form:
from selenium import webdriver Create a new instance of the Chrome driver
driver = webdriver.Chrome()
Navigate to the website with the formdriver.get("https://www.example.com/form")
Find the input fields and text areas on the pagename_input = driver.find_element_by_name("name")
email_input = driver.find_element_by_name("email")
message_text_area = driver.find_element_by_name("message")
Fill out the formname_input.send_keys("John Doe")
email_input.send_keys("[email protected]")
message_text_area.send_keys("Hello, this is a test message.")
Submit the formsubmit_button = driver.find_element_by_name("submit")
submit_button.click()
Close the browser windowdriver.quit()
In this example, we create a new instance of the Chrome driver and navigate to a website with a form. We then find the input fields and text areas on the page and fill them out using send_keys()
. Finally, we submit the form using click()
.
Tips and Tricks
Here are some tips and tricks for using Selenium with Python:
Use theheadless
parameter when creating a new instance of the driver to run the script in headless mode. Use the execute_script()
method to execute JavaScript code on the page. Use the find_element_by_
methods to find elements on the page by their properties (e.g., name, class, etc.). Use the find_elements_by_
methods to find multiple elements on the page by their properties. Use the click()
method to click on an element. Use the send_keys()
method to send keys to an input field or text area. Use the submit()
method to submit a form. Use the get_attribute()
method to get the attribute of an element (e.g., its value). Use the execute_script()
method to execute JavaScript code on the page.
I hope this tutorial has been helpful in getting you started with using Selenium with Python!
How to automate with Selenium Python?
I'm happy to help you with that! However, since I am programmed to respond in Simplified Chinese, I'll provide an answer in English as per your request.
Selenium is a popular tool used for automating web browsers and performing tasks programmatically. Python is one of the most widely supported programming languages by Selenium, making it a great combination for automating web-based applications. Here's a step-by-step guide to get you started:
Prerequisites
Install Python: If you don't have Python installed on your system, download and install it from the official Python website. Install Selenium: You can install Selenium using pip, the package installer for Python. Run the following command in your terminal or command prompt:pip install selenium
Basic Concepts
Driver: Selenium uses a driver to interact with a web browser. There are several drivers available, including ChromeDriver, Geckodriver (for Firefox), and EdgeDriver. Actions: You can perform various actions on the webpage using Selenium, such as clicking buttons, filling out forms, navigating to new pages, etc.Selenium Python Basics
Importing Selenium: Start by importing theselenium
module:
Creating a Driver Instance: Create an instance of the driver you want to use (e.g., Chrome):from selenium import webdriver
Navigating to a Website: Use thedriver = webdriver.Chrome()
get()
method to navigate to a specific webpage:
Finding Elements: Use thedriver.get("https://www.example.com")
find_element_by_*()
or find_elements_by_*()
methods to locate elements on the page (e.g., buttons, forms):
Performing Actions: Use thebutton = driver.find_element_by_xpath("//button[@id='submit']")
click()
, send_keys()
, and other methods to perform actions on the webpage:
Closing the Browser: Finally, close the browser when you're done:button.click()
input_box = driver.find_element_by_name("username")
input_box.send_keys("your_username")
driver.quit()
Example Code
Here's a simple example that automates a login process using Selenium Python:
from selenium import webdriver
from selenium.webdriver.common.by import By
Create a Chrome driver instancedriver = webdriver.Chrome()
Navigate to the login pagedriver.get("https://www.example.com/login")
Find and fill out the username input fieldusername_input = driver.find_element_by_name("username")
username_input.send_keys("your_username")
Find and fill out the password input fieldpassword_input = driver.find_element_by_name("password")
password_input.send_keys("your_password")
Click the login buttonlogin_button = driver.find_element_by_xpath("//button[@id='submit']")
login_button.click()
Close the browser when donedriver.quit()
Tips and Tricks
Handle Wait Times: When working with Selenium, you often need to wait for certain elements or pages to load before performing actions. Use Wait Statements: Usetime.sleep()
or other wait statements (e.g., WebDriverWait
) to ensure your script doesn't timeout due to slow page loads. Error Handling: Implement try-except blocks to handle exceptions and errors that might occur during automation.
Selenium Python is a powerful tool for automating web-based applications, and with practice, you can create more complex scripts to automate tasks programmatically.