Python read json
Python read json
I'd be happy to explain how to use Python to read a JSON file!
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write. It's a popular choice for exchanging data between web servers, web applications, and mobile apps.
Python has a built-in module called json
that allows you to easily work with JSON files. Here are the basic steps:
json
module: At the top of your Python script, add the following line:
import json
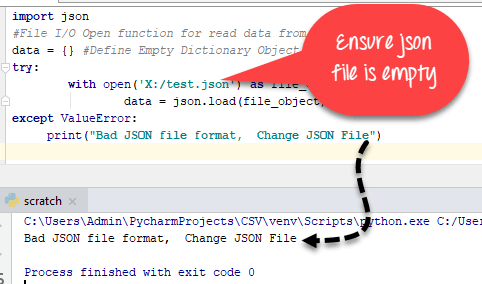
Loading the JSON file: Use the json.load()
function to load the JSON file into a Python object (like a dictionary or list). For example:
with open('path_to_your_json_file.json') as f:
data = json.load(f)
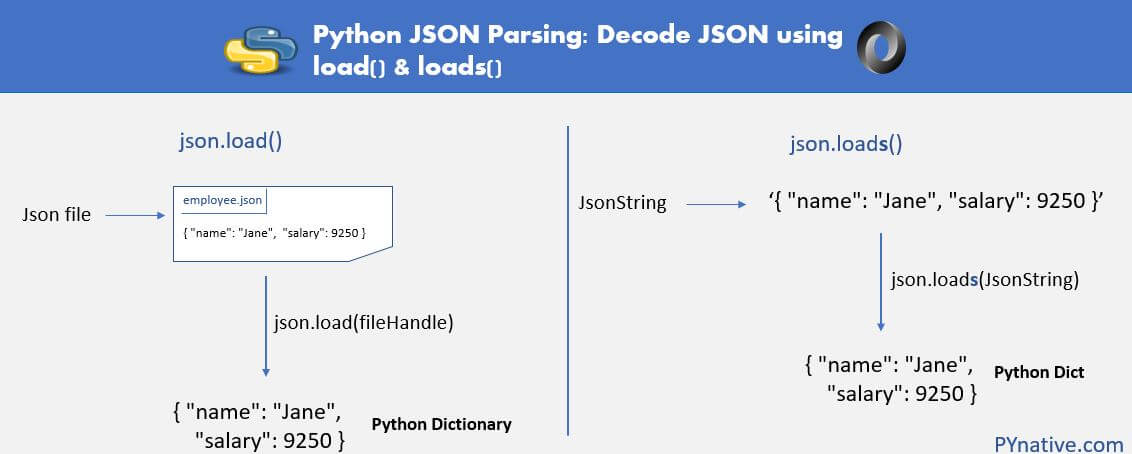
Working with the JSON data: Now that you have loaded the JSON data, you can access its contents using standard Python syntax. Here are a few examples of what you might do: Print out all the keys in the JSON object: print(data.keys())
Get the value associated with a specific key: print(data['specific_key'])
Loop over the items in an array: for item in data['array']: print(item)
Saving changes back to the JSON file: If you've made changes to the JSON data, you can save those changes back to the original file using the json.dump()
function:
with open('path_to_your_json_file.json', 'w') as f:
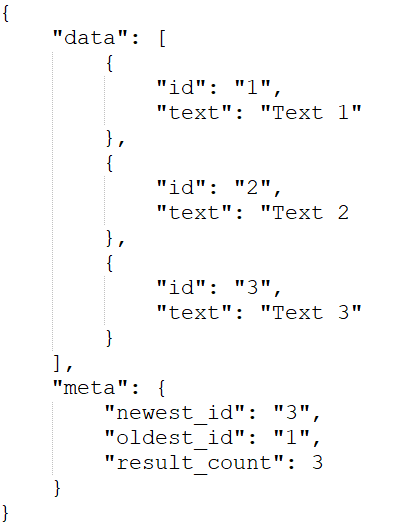
json.dump(data, f)
Some additional notes:
If your JSON file contains nested objects or arrays, Python will automatically convert them into native Python types (like dictionaries and lists). Thejson
module is smart enough to handle many different types of data in your JSON file, including strings, numbers, booleans, null values, and even dates. You can use the json.load()
function with a string instead of a file object if you have the JSON data already stored as a string.
Here's an example of what this might look like in a complete Python script:
import json
def load_json_file():
Load the JSON file
with open('data.json') as f:
data = json.load(f)
Print out all the keys in the JSON object
print("Keys:", data.keys())
Get the value associated with a specific key
specific_key_value = data['specific_key']
print("Value of 'specific_key':", specific_key_value)
Loop over the items in an array
for item in data['array']:
print(item)
def save_changes_to_json_file(data):
Save the changes back to the JSON file
with open('data.json', 'w') as f:
json.dump(data, f)
Example usage:
if name == "main":
load_json_file()
new_data = {'new_key': 'new_value'} # Create a new dictionary to add to the JSON data
save_changes_to_json_file(new_data)
In this script, we import the json
module and define two functions: one for loading the JSON file, and another for saving changes back to the JSON file. We then use these functions in an example usage section at the end of the script.
I hope this helps! Let me know if you have any questions or if there's anything else I can do to help.
How do you read in Python?
I'll respond in English, as per your request.
Python is a versatile programming language that offers several ways to read data from various sources. Here are some of the most common methods:
Reading from Files: Python provides built-in support for reading and writing files using theopen()
function. You can specify the file mode ('r'
for read, 'w'
for write, or 'a'
for append) and the encoding (e.g., 'utf-8'
) when opening a file.
Example:
Reading from Standard Input: Python also allows you to read input from the standard input stream using thewith open('example.txt', 'r') as file:
contents = file.read()
print(contents)
input()
function. This is particularly useful for command-line interfaces or when reading user input.
Example:
Reading JSON Data: Python's built-inuser_input = input("Enter your name: ")
print("Hello, " + user_input)
json
module enables you to read JSON-formatted data from a file or string.
Example:
Reading CSV Data: Python'simport json
data = '{"name": "John", "age": 30}'
data_dict = json.loads(data)
print(data_dict) # {'name': 'John', 'age': 30}
csv
module provides functions for reading and writing comma-separated values (CSV).
Example:
Reading XML Data: Python'simport csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
data = list(reader)
print(data) # [['John', '30'], ['Jane', '25']]
xml.etree.ElementTree
module allows you to read and parse XML files.
Example:
Reading from Databases: Python has various libraries for interacting with databases, such as SQLite, MySQL, PostgreSQL, and more.import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
print(root) #
Example (using the sqlite3
library):
Reading from the Internet: Python'simport sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM people")
rows = cursor.fetchall()
print(rows) # [(1, 'John', 30), (2, 'Jane', 25)]
requests
library enables you to make HTTP requests and read data from web pages or APIs.
Example:
import requests
response = requests.get('https://example.com/api/data')
data = response.json()
print(data) # {'results': [{'name': 'John', 'age': 30}, {'name': 'Jane', 'age': 25}]}
These are just a few examples of how you can read data in Python. The choice of method depends on the specific requirements of your project and the type of data you're working with.