Python threading timer example
Python threading timer example
Here's a basic example of using Python's threading
module to create a timer that prints out messages at regular intervals:
import threading
import time
class Timer:
def init(self):
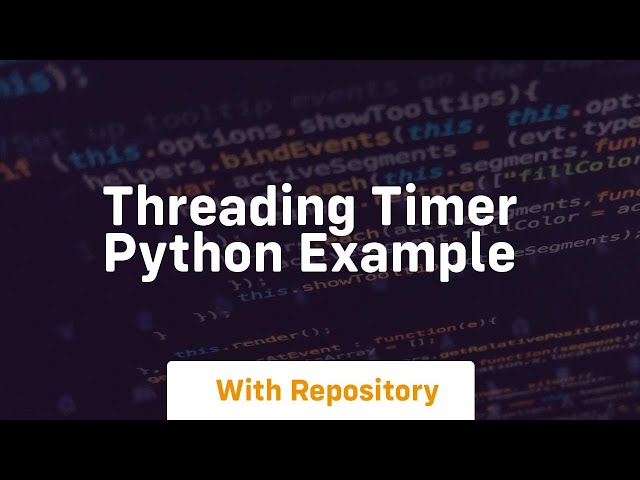
self.running = False
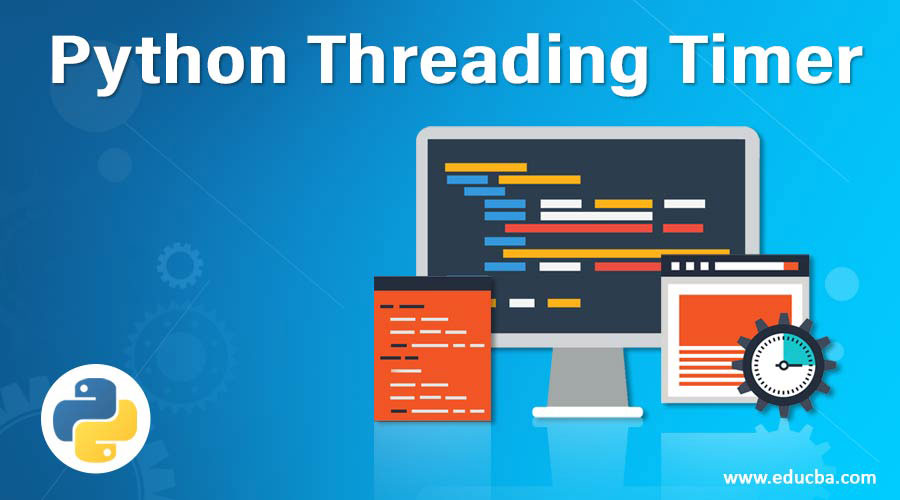
self.lock = threading.Lock()
def start(self):
if not self.running:
self.running = True
self.thread = threading.Thread(target=self._timer)
self.thread.start()
else:
print("Timer is already running!")
def stop(self):
if self.running:
self.running = False
self.thread.join()
else:
print("Timer is not running!")
def _timer(self):
while self.running:
with self.lock:
print(time.strftime("%H:%M:%S", time.localtime()))
time.sleep(1)
def main():
timer = Timer()
try:
while True:
command = input("Enter 'start' to start the timer, or 'stop' to stop it: ")
if command == "start":
timer.start()
elif command == "stop":
timer.stop()
else:
print("Invalid command! Try again.")
except KeyboardInterrupt:
print("nStopping the timer...")
timer.stop()
if name == "main":
main()
To use this code, simply run it and enter 'start' to start the timer. The timer will then print out the current time every second. You can stop the timer by entering 'stop'.
The Timer
class itself is relatively simple: it maintains a boolean flag indicating whether the timer is currently running (running
), as well as a lock (lock
) that you can use to synchronize access to the timer's state.
The start()
method sets the running
flag to True
, starts a new thread running the _timer()
method, and returns.
The stop()
method sets the running
flag to False
, waits for any existing timer events to complete (thread.join()
), and then returns.
In the _timer()
method itself, we loop until running
is set to False
. At each iteration, we grab the lock, print out the current time, release the lock, and sleep for 1 second.
Finally, in the main()
function, we create a new Timer
object, enter an infinite loop where we repeatedly prompt the user for input (input()
) and dispatch commands to start or stop the timer based on what they type.
This code illustrates how you can use Python's threading
module to create simple multithreaded applications, like this timer.
How do you add a delay in Python threading?
In Python, you can add delays using the time module or by utilizing threads and locks with synchronization mechanisms like event objects from the threading module.
Let's look at each approach individually:
Time Module
To add a delay without multithreading, you can use the sleep function provided by the time module. This function is used to pause the execution of a program for a specified amount of time.
Here's an example:
import time
def delayed_function():
print("Delayed function started")
time.sleep(5) # Wait for 5 seconds
print("Delayed function finished")
delayed_function()
In this code, the delayed_function will pause its execution for 5 seconds and then continue with the rest of the program. The sleep function is a blocking call that means your program will pause until the specified time has passed.
Threading Module
To add delays in multiple threads, you can use the threading module along with event objects. An Event object is used to wait until the occurrence of an event or after a certain delay.
Here's how you can use it:
import threading
import time
class MyThread(threading.Thread):
def init(self):
super().init()
self.event = threading.Event()
def run(self):
print("My thread started")
while True:
self.event.wait() # Wait for the event
if not self.event.is_set(): # Check if the event is set
break # If it's set, break the loop and terminate the thread
print("My thread finished")
def delay(self):
time.sleep(5) # Delay by 5 seconds
self.event.set() # Set the event
my_thread = MyThread()
my_thread.start()
my_thread.delay()
In this code, the run function of the MyThread class will keep waiting for an event until it's set. The delay function sets the event after a certain time (in this case, 5 seconds). This way, you can add delays to multiple threads without using a separate thread or process.
Asyncio Module
You can also use asyncio library in Python which has support for coroutines and async/await syntax. You can create an asynchronous function and schedule it with asyncio.sleep() method.
Here's how you can do this:
import asyncio
async def delayed_function():
print("Delayed function started")
await asyncio.sleep(5) # Wait for 5 seconds
print("Delayed function finished")
asyncio.run(delayed_function())
This code will run the delayed_function with a delay of 5 seconds. The sleep() method is an asynchronous operation that allows your program to execute other tasks or events during this time.
Conclusion
In conclusion, you can add delays in Python using time module for single-thread execution and threading module for multithreading. You can also use asyncio library if you want to add delay to a coroutine function.