python class(object) vs class
python class(object) vs class
I'd be happy to help you with that!
In Python, the keywords class
and def
are used to define a new object or function respectively. While both terms might seem similar at first glance, they serve distinct purposes in Python programming.
Class (Object)
A class in Python is essentially a blueprint for creating objects. Think of it as a cookie cutter that shapes the characteristics of an object when you create instances from it. In other words, a class defines the attributes and methods (actions) that an object can possess. When you define a class, you are specifying the fundamental structure of an object.
Here's a basic example:
class Dog:
def init(self, name, age):
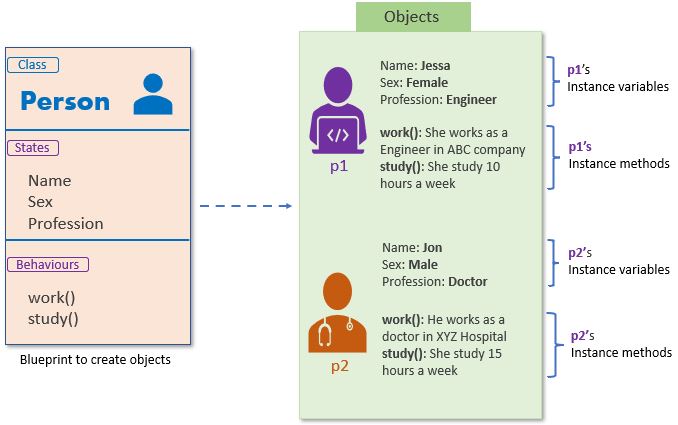
self.name = name
self.age = age
def bark(self):
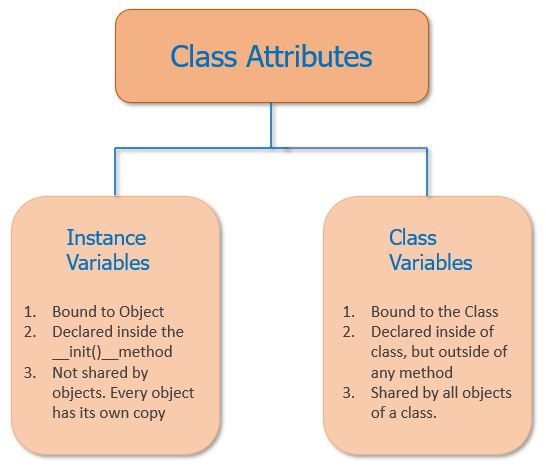
print("Woof!")
my_dog = Dog("Fido", 3)
print(my_dog.name) # prints "Fido"
print(my_dog.age) # prints 3
my_dog.bark() # outputs "Woof!"
In this example, Dog
is a class that has two attributes (name
and age
) and one method (bark
). When we create an instance of the Dog
class, we're creating a new object called my_dog
.
Class (Definition)
On the other hand, when you use the keyword class
to define a class in Python, you are essentially defining a function-like construct that can be used to group together related functions or methods.
Here's an example:
class Counter:
def init(self):
self.count = 0
def increment(self):
self.count += 1
counter = Counter()
print(counter.count) # prints 0
counter.increment()
print(counter.count) # prints 1
In this case, Counter
is a class that has two methods (__init__
and increment
). When we create an instance of the Counter
class, we're creating an object called counter
.
Key differences
Now, here are the key differences between these two uses of the keyword class
:
In summary, Python's class
keyword has two distinct meanings: as an object, which defines a blueprint for creating objects with attributes and methods; and as a definition, which groups related functions or methods together. Understanding these differences is crucial to effectively using classes in your Python programming endeavors!
How many objects can be created from a class Python?
I'd be happy to answer your question!
In Python, when you define a class using the class
keyword, it's called a blueprint for creating objects. By default, an object (an instance of a class) is created when you use the ()
operator on the class name, followed by any required arguments. This process is called instantiation.
Now, let's dive into the answer!
According to Python's official documentation, "A class can be used to create multiple objects." In other words, you can create as many instances of a class as you want, and each instance will have its own set of attributes (data) and methods (functions).
In practice, this means that if you define a class called Car
, you can create multiple Car
objects, each with its own unique characteristics, such as color, make, model, year, and so on. For example:
class Car:
def init(self, color, make, model):
self.color = color
self.make = make
self.model = model
Create the first car object
car1 = Car("Red", "Toyota", "Corolla")
print(car1.color) # Output: Red
print(car1.make) # Output: Toyota
print(car1.model) # Output: Corolla
Create the second car object (different attributes)
car2 = Car("Blue", "Honda", "Civic")
print(car2.color) # Output: Blue
print(car2.make) # Output: Honda
print(car2.model) # Output: Civic
And so on...
As you can see, each Car
object has its own set of attributes (color, make, model), and you can create multiple objects with different attribute values.
In Python, there is no theoretical limit to the number of objects that can be created from a class. You can create as many instances as your system's memory allows!
To illustrate this further, imagine a real-world scenario where you're creating Employee
objects in a company database:
class Employee:
def init(self, name, department, salary):
self.name = name
self.department = department
self.salary = salary
Create the first employee object
emp1 = Employee("John", "Sales", 50000)
print(emp1.name) # Output: John
print(emp1.department) # Output: Sales
print(emp1.salary) # Output: 50000
Create the second employee object (different attributes)
emp2 = Employee("Jane", "Marketing", 60000)
print(emp2.name) # Output: Jane
print(emp2.department) # Output: Marketing
print(emp2.salary) # Output: 60000
And so on...
In this example, you could create hundreds or even thousands of Employee
objects, each with unique attributes (name, department, salary).
So, to answer your question, the number of objects that can be created from a class in Python is theoretically unlimited, as long as there's enough memory available!
I hope this explanation helped clarify things for you!