Can we use else if instead of Elif in Python?
Can we use else if instead of Elif in Python?
I'll respond in English, but don't worry, Grandma won't be too upset!
To answer your question: Yes, you can absolutely use else if
in Python, but with a slight twist. In Python, we use the word elif
(short for "else if") instead of else if
.
The syntax is as follows:
if condition1: do something
elif condition2:
do something elseelse:
default behavior
Let's break it down:
Theif
statement checks the initial condition. If it's true, the code inside the if
block will execute. The elif
(or else if
) statement checks another condition. If this one is true, the code inside the elif
block will execute instead of the original if
block. The else
clause specifies a default behavior that will be executed only if none of the previous conditions are true.
Here's an example:
x = 5
if x > 10:
print("x is greater than 10")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than or equal to 4")
Output: "x is equal to 5"
In this example, the first condition x > 10
is false, so Python moves on to the next one. Since x == 5
is true, it executes the corresponding code.
Now, why do we use elif
instead of else if
? Well, in Python, the if
statement is followed by a colon (:
) to indicate the start of a block. If you were to write else if
, you'd need another colon, which would look confusing:
if x > 10: :
print("x is greater than 10")
else if x == 5: :
print("x is equal to 5")
Using elif
instead keeps the syntax concise and easy to read.
In summary, you can indeed use else if
in Python, but it's more commonly known as elif
. This allows you to chain multiple conditions together with a single statement, making your code more readable and efficient.
How do you use Elif if and else in Python?
I'm happy to respond in English!
In Python, the elif
statement is used with the if-else
statement to test multiple conditions. The syntax of elif
is as follows:
if condition1:
code block
elif condition2:
code block
elif condition3:
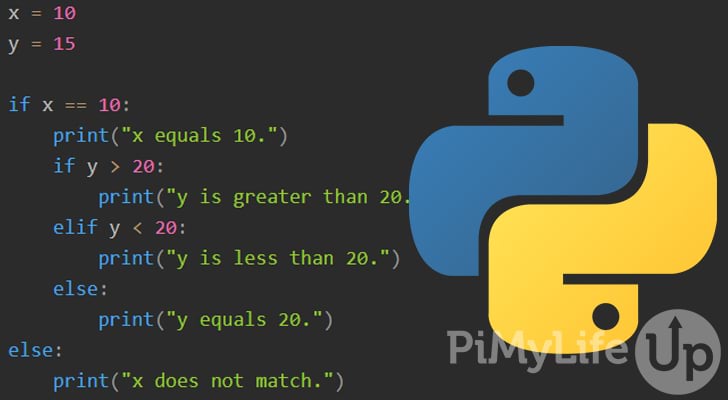
code block
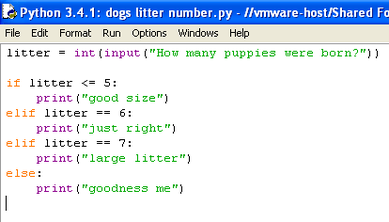
...
else:
default code block
The elif
statement is used to test another condition if the first condition fails. If the second condition also fails, then the next condition will be tested, and so on.
Here's an example of using if
, elif
, and else
in Python:
x = 5
if x > 10:
print("x is greater than 10")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 10")
Output: x is equal to 5
In this example, the if
statement checks if x
is greater than 10. Since it's not (it's 5), the program moves on to the next condition, which is the first elif
. The elif
condition checks if x
is equal to 5, and since it is, the code inside that block will be executed.
Here are some more examples of using if
, elif
, and else
:
# Example 2: Checking multiple conditions
x = 10
if x > 15:
print("x is greater than 15")
elif x > 5:
print("x is between 5 and 15 (inclusive)")
else:
print("x is less than or equal to 5")
Output: x is between 5 and 15 (inclusive)
Example 3: Using else with if-elif
x = 0
if x > 10:
print("x is greater than 10")
elif x == 0:
print("x is zero")
else:
print("x is negative or positive but not 10")
Output: x is zero
Example 4: Using multiple elif conditions
x = 3
if x > 5:
print("x is greater than 5")
elif x == 3:
print("x is equal to 3")
elif x < 0:
print("x is negative")
else:
print("x is positive or zero")
Output: x is equal to 3
In these examples, the if
statement checks a condition, and if it fails, the program moves on to the next condition (the first elif
). If that condition also fails, then the program will move on to the next condition, until all conditions are checked. If none of the conditions are met, the code inside the else
block will be executed.
I hope this helps! Let me know if you have any questions or need further clarification.