How to write an if statement in Python?
How to write an if statement in Python?
I'm glad you're interested in learning Python! Writing an "if" statement in Python is a fundamental concept in programming, and I'd be happy to explain it to you.
The "if" statement in Python is used to execute a block of code if a certain condition is met. The basic syntax for an "if" statement is as follows:
if condition:
code to be executed if the condition is True
Here, condition
can be any valid Python expression that evaluates to a boolean value (i.e., either True or False). If the condition
is true, then the indented block of code will be executed. If the condition
is false, the block of code will not be executed.
Let's take a simple example:
x = 5
if x > 3:
print("x is greater than 3")
In this example, we first assign the value 5 to the variable x
. Then, we use an "if" statement to check if x
is greater than 3. If x
is indeed greater than 3 (which it is), then the indented print statement will be executed, printing out the message "x is greater than 3".
Now, let's take a more complex example:
x = 2
if x > 3 and y == 4:
print("Both conditions are True")
In this case, we have two conditions: x
is greater than 3, and y
is equal to 4. If both conditions are true (which they aren't), then the indented block of code will be executed, printing out the message "Both conditions are True".
Note that if you want to execute different blocks of code depending on whether the condition is true or false, you can use an "else" statement:
x = 2
if x > 3:
print("x is greater than 3")
else:
print("x is less than or equal to 3")
In this example, if x
is greater than 3 (which it isn't), then the first block of code will be executed. If x
is not greater than 3, then the second block of code will be executed.
Finally, let's talk about "elif" statements:
x = 2
if x > 5:
print("x is greater than 5")
elif x == 3:
print("x is equal to 3")
else:
print("x is less than 3")
In this example, we have three conditions. If x
is greater than 5 (which it isn't), then the first block of code will be executed. If x
is not greater than 5 and is equal to 3 (which it is), then the second block of code will be executed. If x
is neither greater than 5 nor equal to 3, then the third block of code will be executed.
So there you have it! "If" statements in Python are used to execute different blocks of code based on certain conditions. With a little practice, you'll become proficient in writing your own if statements and tackling more complex programming tasks.
If else statement in python example w3schools
I'll respond in English as requested!
W3Schools is a great resource for learning web development, including programming languages like Python. Let's dive into an example of using the if...else
statement in Python.
Example: Conditional Statement with if...else
In this example, we'll create a simple program that asks the user to input their age and then prints out a message based on their age:
age = int(input("Enter your age: "))
if age >= 18:
print("You are an adult!")
else:
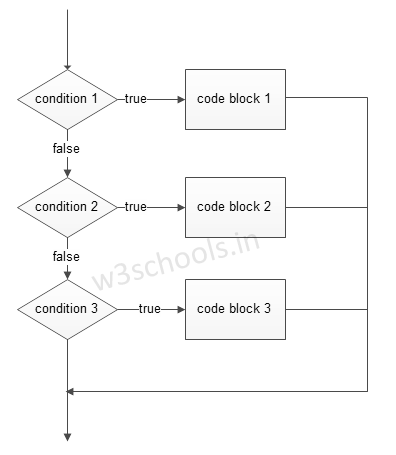
print("You are a minor!")
Here's how this code works:
Theinput()
function is used to get the user's input (in this case, their age). The int()
function converts the input into an integer value. The if
statement checks if the age is 18 or older using the >=
operator (which means "greater than or equal to"). If the condition is true (age >= 18
), the code prints out the message "You are an adult!". If the condition is false (i.e., the user's age is less than 18), the code jumps to the else
block and prints out the message "You are a minor!".
Output:
When you run this program, it will ask you to input your age. If you enter an age of 17 or less, it will print:
You are a minor!
If you enter an age of 18 or older, it will print:
You are an adult!
Key Takeaways:
Theif
statement is used to check a condition and execute code if the condition is true. The else
block is used when the condition is false. The int()
function is used to convert user input into an integer value.
This simple example demonstrates the basic syntax of an if...else
statement in Python, which can be useful for making decisions based on conditions or inputs. W3Schools has many more examples and tutorials on this topic if you want to learn more!